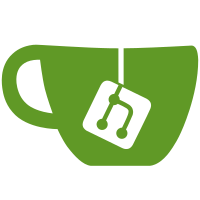
Co-authored-by: Sebastian Piotrowski <sebpio@st.amu.edu.pl> Co-authored-by: Marcin Matoga <marmat35@st.amu.edu.pl> Co-authored-by: Ladislaus3III <Ladislaus3III@users.noreply.github.com>
106 lines
2.9 KiB
Python
106 lines
2.9 KiB
Python
import numpy as np
|
|
import math
|
|
import random
|
|
from geneticalgorithm import geneticalgorithm as ga
|
|
from astar2 import *
|
|
|
|
|
|
class Mine():
|
|
def __init__(self, row, column):
|
|
self.row = row
|
|
self.columnt = column
|
|
|
|
|
|
|
|
|
|
|
|
class GeneticalWithLib():
|
|
|
|
Miasta = [[0, 130, 180, 300],
|
|
[130, 0, 320, 350],
|
|
[180, 320, 0, 360],
|
|
[300, 350, 360, 0]]
|
|
#Miasta = [[0, 2, 6, 3, 7],
|
|
# [2, 0, 6, 4, 8],
|
|
# [6, 6, 0, 5, 8],
|
|
# [3, 4, 5, 0, 9],
|
|
# [7, 8, 8, 9, 0]]
|
|
|
|
self.mine_points = set()
|
|
|
|
|
|
def getCoordinates(self):
|
|
temp_map = [list(item) for item in SweeperAgent.loadMap('genetic_maps/map1.txt')]
|
|
|
|
a_row = 0
|
|
a_column = 0
|
|
|
|
for row in range(MAP_SIZE):
|
|
for column, pos in enumerate(temp_map[row]):
|
|
if pos == "m" :
|
|
a_row = row
|
|
a_column = column
|
|
location = tuple([a_row, a_column])
|
|
self.mine_points.add(location)
|
|
|
|
def setDistance(self):
|
|
pass
|
|
|
|
|
|
def fillDistanceMatrix(self):
|
|
pass
|
|
|
|
|
|
|
|
def f(X):
|
|
for i in range(len(Miasta[0])):
|
|
if X[i] not in Miasta[i]:
|
|
return np.sum(X)+12000
|
|
for i in range(len(Miasta[0])):
|
|
if i == 0:
|
|
if (X[0]-X[len(Miasta[0])-1]) == 0:
|
|
return np.sum(X)+12000
|
|
else:
|
|
if abs(X[i]-X[i-1]) == 0:
|
|
return np.sum(X)+12000
|
|
if i != len(Miasta[0])-1:
|
|
if abs(X[i+1]-X[i-1]) == 0:
|
|
return np.sum(X)+12000
|
|
var = []
|
|
for j in range(len(Miasta[0])):
|
|
var.append(0)
|
|
for i in range(len(Miasta[0])):
|
|
var[Miasta[i].index(X[i])] += 1
|
|
for s in var:
|
|
if s != 1:
|
|
return np.sum(X)+9000
|
|
return np.sum(X)
|
|
|
|
varbound = np.array([[130,300],
|
|
[130,350],
|
|
[180,360],
|
|
[300,360]])
|
|
|
|
#varbound = np.array([[2,7],
|
|
# [2,8],
|
|
# [5,8],
|
|
# [4,9],
|
|
# [7,9]])
|
|
|
|
algorithm_param = {'max_num_iteration': 3000,\
|
|
'population_size':100,\
|
|
'mutation_probability':0.1,\
|
|
'elit_ratio': 0.01,\
|
|
'crossover_probability': 0.5,\
|
|
'parents_portion': 0.3,\
|
|
'crossover_type':'uniform',\
|
|
'max_iteration_without_improv':None}
|
|
|
|
model=ga(function=f,\
|
|
dimension=len(Miasta[0]),\
|
|
variable_type='int',\
|
|
variable_boundaries=varbound,\
|
|
algorithm_parameters=algorithm_param)
|
|
|
|
model.run()
|
|
#print(f([180, 350, 180, 350])) |