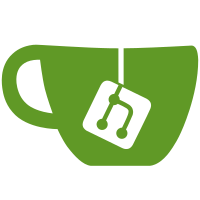
Co-authored-by: Marcin Matoga <marmat35@st.amu.edu.pl> Co-authored-by: Sebastian Piotrowski <sebpio@st.amu.edu.pl>
368 lines
14 KiB
Python
368 lines
14 KiB
Python
import pygame as pg
|
|
import sys
|
|
import ctypes
|
|
from os import path
|
|
|
|
from matplotlib import pyplot
|
|
from sklearn import datasets
|
|
from sklearn.tree import DecisionTreeClassifier
|
|
from sklearn import tree
|
|
|
|
from maze import *
|
|
from grid import *
|
|
from settings import *
|
|
from sprites import *
|
|
from graphsearch import *
|
|
from astar2 import *
|
|
from pizza import *
|
|
from learning import *
|
|
from nn import *
|
|
from genetic import *
|
|
#from genetic3 import *
|
|
#from csv_writer import *
|
|
|
|
|
|
|
|
class Game:
|
|
def __init__(self):
|
|
pg.init()
|
|
self.screen = pg.display.set_mode((WIDTH, HEIGHT))
|
|
pg.display.set_caption(TITLE)
|
|
self.clock = pg.time.Clock()
|
|
pg.key.set_repeat(500, 100)
|
|
self.load_data()
|
|
self.wentyl_bezpieczenstwa = 0
|
|
self.minefield = False
|
|
self.minefield_start_map = "Looper_maps/start_map"
|
|
self.orientation_save = "default"
|
|
# ExampleGenerator.generate(ExampleGenerator)
|
|
|
|
def load_data(self, map_name = "main_map.txt"):
|
|
game_folder = path.dirname(__file__)
|
|
self.map_data = []
|
|
with open(path.join(game_folder, map_name), 'rt') as f:
|
|
for line in f:
|
|
self.map_data.append(line)
|
|
|
|
|
|
def new(self):
|
|
# initialize all variables and do all the setup for a new game
|
|
#glebokosc rozmiar masa moc szkodliwosc zabawka stan teledysk
|
|
# x0 = glebokosc x1 = rozmiar x2 = masa x3 = moc x4 = szkodliwosc x5 = zabawka x6 = stan x7 = teledysk
|
|
# oflagowac, zdetonowac, sprzedac na allegro, sprzedac na czarnym rynku, obejrzec
|
|
self.all_sprites = pg.sprite.Group()
|
|
self.walls = pg.sprite.Group()
|
|
self.mines = pg.sprite.Group()
|
|
self.puddles = pg.sprite.Group()
|
|
for row, tiles in enumerate(self.map_data):
|
|
for col, tile in enumerate(tiles):
|
|
if tile == '2':
|
|
Mine(self, col, row)
|
|
Mine.set_parameters(Mine,18,32,6,7,0,1,0,0)
|
|
if tile == '3':
|
|
Bomb(self, col, row)
|
|
if tile == '4':
|
|
Grenade(self, col, row)
|
|
if tile == "#":
|
|
Wall(self, col, row)
|
|
if tile == 'p':
|
|
Puddle(self, col, row)
|
|
if tile == '>':
|
|
self.player = Player(self, col, row, Direction.Right.name)
|
|
if tile == '^':
|
|
self.player = Player(self, col, row, Direction.Up.name)
|
|
if tile == '<':
|
|
self.player = Player(self, col, row, Direction.Left.name)
|
|
if tile == 'v':
|
|
self.player = Player(self, col, row, Direction.Down.name)
|
|
|
|
|
|
def run(self):
|
|
# game loop - set self.playing = False to end the game
|
|
self.playing = True
|
|
while self.playing:
|
|
self.events()
|
|
self.update()
|
|
self.draw()
|
|
|
|
def quit(self):
|
|
pg.quit()
|
|
sys.exit()
|
|
|
|
def update(self):
|
|
# update portion of the game loop
|
|
self.all_sprites.update()
|
|
|
|
def draw_grid(self):
|
|
for x in range(0, WIDTH, TILESIZE):
|
|
pg.draw.line(self.screen, LIGHTGREY, (x, 0), (x, HEIGHT))
|
|
for y in range(0, HEIGHT, TILESIZE):
|
|
pg.draw.line(self.screen, LIGHTGREY, (0, y), (WIDTH, y))
|
|
|
|
def draw(self):
|
|
self.screen.fill(BGCOLOR)
|
|
self.draw_grid()
|
|
self.all_sprites.draw(self.screen)
|
|
pg.display.flip()
|
|
|
|
def events(self):
|
|
# catch all events here
|
|
|
|
for event in pg.event.get():
|
|
if event.type == pg.QUIT:
|
|
self.quit()
|
|
if event.type == pg.KEYDOWN:
|
|
if event.key == pg.K_ESCAPE:
|
|
self.quit()
|
|
if event.key == pg.K_LEFT:
|
|
self.player.move(dx=0, move='Left')
|
|
if event.key == pg.K_RIGHT:
|
|
self.player.move(dx=0, move='Right')
|
|
if event.key == pg.K_UP:
|
|
self.player.move(dy=0, move='Forward')
|
|
if event.key == pg.K_F2 and self.wentyl_bezpieczenstwa == 0:
|
|
|
|
self.player.maze.run()
|
|
self.player.parse_maze_moves()
|
|
self.i_like_to_move_it()
|
|
self.wentyl_bezpieczenstwa = 1
|
|
if event.key == pg.K_F3 and self.wentyl_bezpieczenstwa == 0:
|
|
pg.event.clear()
|
|
player_moves = BFS.run()
|
|
self.graph_move(player_moves)
|
|
self.wentyl_bezpieczenstwa = 1
|
|
if event.key == pg.K_F4 and self.wentyl_bezpieczenstwa == 0:
|
|
pg.event.clear()
|
|
#print("test1")
|
|
agent = SweeperAgent()
|
|
player_moves = SweeperAgent.run(agent)
|
|
self.graph_move(player_moves)
|
|
self.wentyl_bezpieczenstwa = 1
|
|
|
|
# Test.run()
|
|
if event.key == pg.K_F5:
|
|
self.player.decision_tree_learning()
|
|
#print("lol xD")
|
|
pg.event.clear()
|
|
if event.key == pg.K_F6 and self.wentyl_bezpieczenstwa == 0:
|
|
#LabelSetter.set_labels()
|
|
self.player.ai_mode = "tree"
|
|
agent = SweeperAgent()
|
|
player_moves = SweeperAgent.run(agent)
|
|
self.graph_move(player_moves)
|
|
self.wentyl_bezpieczenstwa = 1
|
|
if event.key == pg.K_F7 and self.wentyl_bezpieczenstwa == 0:
|
|
#net_runner = NetRunner()
|
|
#net_runner.prepare_data()
|
|
self.player.ai_mode = "neural"
|
|
agent = SweeperAgent()
|
|
player_moves = SweeperAgent.run(agent)
|
|
self.graph_move(player_moves)
|
|
self.wentyl_bezpieczenstwa = 1
|
|
if event.key == pg.K_F8 and self.wentyl_bezpieczenstwa == 0:
|
|
#print("pressed")
|
|
genetic_runner = Genetic()
|
|
genetic_runner.run()
|
|
self.wentyl_bezpieczenstwa = 1
|
|
if event.key == pg.K_F9 and self.wentyl_bezpieczenstwa == 0:
|
|
#genetic_lib_runner = GeneticalWithLib()
|
|
#genetic_lib_runner.run()
|
|
|
|
#print(str(self.player.get_direction()).upper())
|
|
|
|
self.wentyl_bezpieczenstwa = 1
|
|
if event.key == pg.K_F10 and self.wentyl_bezpieczenstwa == 0:
|
|
self.player.ai_mode = "tree"
|
|
self.player.check_bomb()
|
|
self.wentyl_bezpieczenstwa = 1
|
|
if event.key == pg.K_F11 and self.wentyl_bezpieczenstwa == 0:
|
|
self.player.check_bomb_neural()
|
|
self.wentyl_bezpieczenstwa = 1
|
|
if event.key == pg.K_F12 and self.wentyl_bezpieczenstwa == 0:
|
|
g.load_data('Looper_maps/sprite_map')
|
|
g.new()
|
|
g.show_go_screen()
|
|
self.minefield = True
|
|
self.wentyl_bezpieczenstwa = 1
|
|
if event.key == pg.K_F1 and self.wentyl_bezpieczenstwa == 0:
|
|
self.player.reset()
|
|
self.wentyl_bezpieczenstwa = 1
|
|
if self.minefield == True:
|
|
if event.key == pg.K_1 and self.wentyl_bezpieczenstwa == 0:
|
|
agent = SweeperAgent()
|
|
if self.minefield_start_map == "Looper_maps/start_map":
|
|
player_moves = SweeperAgent.run_manual_map(agent, self.minefield_start_map, "Looper_maps/mine1_map")
|
|
else:
|
|
player_moves = SweeperAgent.run_manual_map(agent, self.minefield_start_map, "Looper_maps/mine1_map", orientation=self.orientation_save)
|
|
|
|
self.minefield_start_map = 'Looper_maps/mine1_map'
|
|
self.orientation_save = self.orientation_reverse(str(self.player.get_direction()).upper())
|
|
|
|
|
|
self.graph_move(player_moves)
|
|
|
|
self.wentyl_bezpieczenstwa = 1
|
|
if event.key == pg.K_2 and self.wentyl_bezpieczenstwa == 0:
|
|
agent = SweeperAgent()
|
|
if self.minefield_start_map == "Looper_maps/start_map":
|
|
player_moves = SweeperAgent.run_manual_map(agent, self.minefield_start_map, "Looper_maps/mine2_map")
|
|
else:
|
|
player_moves = SweeperAgent.run_manual_map(agent, self.minefield_start_map, "Looper_maps/mine2_map", orientation=self.orientation_save)
|
|
|
|
self.minefield_start_map = 'Looper_maps/mine2_map'
|
|
self.orientation_save = self.orientation_reverse(str(self.player.get_direction).upper())
|
|
|
|
self.graph_move(player_moves)
|
|
|
|
self.wentyl_bezpieczenstwa = 1
|
|
if event.key == pg.K_3 and self.wentyl_bezpieczenstwa == 0:
|
|
agent = SweeperAgent()
|
|
player_moves = SweeperAgent.run_manual_map(agent, self.minefield_start_map, "Looper_maps/mine3_map")
|
|
self.minefield_start_map = "Looper_maps/mine3_map"
|
|
self.graph_move(player_moves)
|
|
self.wentyl_bezpieczenstwa = 1
|
|
if event.key == pg.K_4 and self.wentyl_bezpieczenstwa == 0:
|
|
agent = SweeperAgent()
|
|
player_moves = SweeperAgent.run_manual_map(agent, self.minefield_start_map, "Looper_maps/mine4_map")
|
|
self.minefield_start_map = "Looper_maps/mine4_map"
|
|
self.graph_move(player_moves)
|
|
self.wentyl_bezpieczenstwa = 1
|
|
if event.key == pg.K_F1 and self.wentyl_bezpieczenstwa == 0:
|
|
self.minefield_start_map = "Looper_maps/start_map"
|
|
self.wentyl_bezpieczenstwa = 1
|
|
|
|
|
|
|
|
|
|
|
|
if event.type == pg.KEYUP:
|
|
if event.key == pg.K_F1:
|
|
self.wentyl_bezpieczenstwa = 0
|
|
if event.key == pg.K_F2:
|
|
self.wentyl_bezpieczenstwa = 0
|
|
if event.key == pg.K_F3:
|
|
self.wentyl_bezpieczenstwa = 0
|
|
if event.key == pg.K_F4:
|
|
self.wentyl_bezpieczenstwa = 0
|
|
if event.key == pg.K_F5:
|
|
self.wentyl_bezpieczenstwa = 0
|
|
if event.key == pg.K_F6:
|
|
self.wentyl_bezpieczenstwa = 0
|
|
if event.key == pg.K_F7:
|
|
self.wentyl_bezpieczenstwa = 0
|
|
if event.key == pg.K_F8:
|
|
self.wentyl_bezpieczenstwa = 0
|
|
if event.key == pg.K_F9:
|
|
self.wentyl_bezpieczenstwa = 0
|
|
if event.key == pg.K_F10:
|
|
self.wentyl_bezpieczenstwa = 0
|
|
if event.key == pg.K_F11:
|
|
self.wentyl_bezpieczenstwa = 0
|
|
if event.key == pg.K_F12:
|
|
self.wentyl_bezpieczenstwa = 0
|
|
if self.minefield == True:
|
|
if event.key == pg.K_1:
|
|
self.wentyl_bezpieczenstwa = 0
|
|
if event.key == pg.K_2:
|
|
self.wentyl_bezpieczenstwa == 0
|
|
if event.key == pg.K_3:
|
|
self.wentyl_bezpieczenstwa == 0
|
|
if event.key == pg.K_4:
|
|
self.wentyl_bezpieczenstwa == 0
|
|
|
|
|
|
def minefield_looping(self):
|
|
pass
|
|
|
|
def orientation_reverse(self, orientation):
|
|
if orientation == 'LEFT':
|
|
return 'RIGHT'
|
|
if orientation == 'RIGHT':
|
|
return 'LEFT'
|
|
if orientation == 'UP':
|
|
return 'DOWN'
|
|
if orientation == 'DOWN':
|
|
return 'UP'
|
|
pass
|
|
|
|
def graph_move(self, moves):
|
|
for i in moves:
|
|
if i == 'Right':
|
|
self.player.move(dx=0, move='Right')
|
|
self.update()
|
|
self.draw()
|
|
pg.time.delay(250)
|
|
if i == 'Left':
|
|
self.player.move(dx=0, move='Left')
|
|
self.update()
|
|
self.draw()
|
|
pg.time.delay(250)
|
|
if i == 'Forward':
|
|
self.player.move(dx=0, move='Forward')
|
|
self.update()
|
|
self.draw()
|
|
pg.time.delay(250)
|
|
|
|
|
|
def i_like_to_move_it(self):
|
|
for i in self.player.moves:
|
|
if i == 'Right':
|
|
self.player.move(dx=1, direction='Right')
|
|
self.update()
|
|
self.draw()
|
|
pg.time.delay(250)
|
|
if i == 'Turn Right':
|
|
self.player.move(0, 0, direction='Right')
|
|
self.update()
|
|
self.draw()
|
|
pg.time.delay(250)
|
|
if i == 'Left':
|
|
self.player.move(dx=-1, direction='Left')
|
|
self.update()
|
|
self.draw()
|
|
pg.time.delay(250)
|
|
if i == 'Turn Left':
|
|
self.player.move(0, 0, direction='Left')
|
|
self.update()
|
|
self.draw()
|
|
pg.time.delay(250)
|
|
if i == 'Down':
|
|
self.player.move(dy=1, direction='Down')
|
|
self.update()
|
|
self.draw()
|
|
pg.time.delay(250)
|
|
if i == 'Turn Down':
|
|
self.player.move(0, 0, direction='Down')
|
|
self.update()
|
|
self.draw()
|
|
pg.time.delay(250)
|
|
if i == 'Up':
|
|
self.player.move(dy=-1, direction='Up')
|
|
self.update()
|
|
self.draw()
|
|
pg.time.delay(250)
|
|
if i == 'Turn Up':
|
|
self.player.move(0, 0, direction='Up')
|
|
self.update()
|
|
self.draw()
|
|
pg.time.delay(250)
|
|
|
|
|
|
def show_start_screen(self):
|
|
pass
|
|
|
|
def show_go_screen(self):
|
|
pass
|
|
|
|
# create the game object
|
|
g = Game()
|
|
g.show_start_screen()
|
|
|
|
#m = Maze()
|
|
#m.run()
|
|
|
|
while True:
|
|
g.new()
|
|
g.run()
|
|
g.show_go_screen()
|