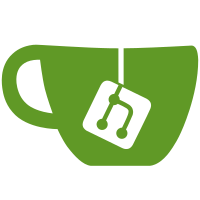
Co-authored-by: Marcin Matoga <marmat35@st.amu.edu.pl> Co-authored-by: Sebastian Piotrowski <sebpio@st.amu.edu.pl> Co-authored-by: Ladislaus3III <Ladislaus3III@users.noreply.github.com>
118 lines
2.5 KiB
Python
118 lines
2.5 KiB
Python
import queue
|
|
from os import path
|
|
|
|
class Maze:
|
|
def __init__(self):
|
|
self.maze = []
|
|
self.moves = ""
|
|
|
|
|
|
|
|
|
|
|
|
def loadMaze(self):
|
|
maze_folder = path.dirname(__file__)
|
|
with open(path.join(maze_folder, 'map.txt'), 'rt') as f:
|
|
for line in f:
|
|
self.maze.append(line)
|
|
return self.maze
|
|
|
|
def printMaze(self, maze, path=""):
|
|
for x, pos in enumerate(maze[0]):
|
|
if pos == "A":
|
|
start = x
|
|
|
|
i = start
|
|
j = 0
|
|
pos = set()
|
|
for move in path:
|
|
if move == "L":
|
|
i -= 1
|
|
|
|
elif move == "R":
|
|
i += 1
|
|
|
|
elif move == "U":
|
|
j -= 1
|
|
|
|
elif move == "D":
|
|
j += 1
|
|
pos.add((j,i))
|
|
|
|
for j, row in enumerate(maze):
|
|
for i, col in enumerate(row):
|
|
if (j, i) in pos:
|
|
print("+ ", end="")
|
|
else:
|
|
print(col + " ", end="")
|
|
print()
|
|
|
|
def valid(self, maze, moves):
|
|
for x, pos in enumerate(maze[0]):
|
|
if pos == "A":
|
|
start = x
|
|
|
|
i = start
|
|
j = 0
|
|
for move in moves:
|
|
if move == "L":
|
|
i -= 1
|
|
|
|
elif move == "R":
|
|
i += 1
|
|
|
|
elif move == "U":
|
|
j -= 1
|
|
|
|
elif move == "D":
|
|
j += 1
|
|
|
|
if not(0 <= i < len(maze[0]) and 0 <= j < len(maze)):
|
|
return False
|
|
|
|
|
|
return True
|
|
|
|
def findEnd(self, maze, moves):
|
|
for x, pos in enumerate(maze[0]):
|
|
if pos == "A":
|
|
start = x
|
|
|
|
i = start
|
|
j = 0
|
|
for move in moves:
|
|
if move == "L":
|
|
i -= 1
|
|
|
|
elif move == "R":
|
|
i += 1
|
|
|
|
elif move == "U":
|
|
j -= 1
|
|
|
|
elif move == "D":
|
|
j += 1
|
|
|
|
if maze[j][i] == "2":
|
|
print("Found: " + moves)
|
|
self.moves = moves
|
|
self.printMaze(maze, moves)
|
|
return True
|
|
|
|
return False
|
|
|
|
def run(self):
|
|
nums = queue.Queue()
|
|
nums.put("")
|
|
add = ""
|
|
self.loadMaze()
|
|
|
|
while not self.findEnd(self.maze, add):
|
|
add = nums.get()
|
|
for j in ["L", "R", "U", "D"]:
|
|
put = add + j
|
|
if self.valid(self.maze, put):
|
|
nums.put(put)
|
|
|
|
|