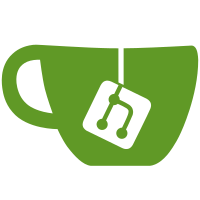
Closes #1640. All Wikibase-dependent parameters, which were previously hard-coded for Wikidata, are now described in a JSON manifest. The manifest is currently constructed by hand, but, in the future, will hopefully be published by each Wikibase instance at a standard location. * setup the manifest framework * add dependency mechanism to scrutinizers & update tests * add json creators to constraint entities * adapt the backend (units tests are to be updated) * remove the call to prepareDependencies() in the constructor * update code according to review feedback * update scrutinizers tests * fix typo & update ConstraintsV1 * log if a scrutinizer is skipped * update versioning handling in the backend * correct the order of "actual" and "expected" for assertEquals method * use regex to check manifest versions * 1. add wikibase-manager.js, wikibase-dialog.js, etc. 2. move dialog/schema-alignment-dialog.js -> schema-alignment.js 3. remove unused schema-alignment-dialog.html 4. change most mentions of "Wikidata" to "Wikibase" * support saving cookies for different Wikibases & fix LoginCommandTest * fix schema related tests * removed unused WikibaseCredentials * include MediaWiki API endpoint in the schema * fetch language codes for different Wikibases * fix lgtm-bot alerts * keep a connection map (MediaWiki API endpoint => Connection) in ConnectionManager * simplify the constraint configurations of the manifest and remove lots of unnecessary code. * add slash to the end of mediawiki.root * add manifest schema and use ajv to validate the manifest * remove JSONP support (Wikibase manifest host should support CORS) * save manifests on manifest update * add unit tests for Manifest * include the exception in logger.error() method to make it easier to debug * include the message of ManifestException when calling respondError * test multiple connections * test no manifest & test invalid manifest * adapt manage-account-dialog.js to support multiple Wikibase connections * update instance/subclass of related translations * beautify import-schema-dialog.html * use "${lang}" variable in the reconciliation service endpoint of the manifest * adapt schema-alignment.js after introducing "${lang}" variable in the reconciliation service endpoint * use WikibaseManager.getSelectedWikibaseApi() in SchemaAlignment._getPropertyType * replace more mentions of "Wikidata" to "Wikibase" * use WikibaseManager.getSelectedWikibaseApi() in previewrenderer.js * support fetching language codes of different Wikibases in the frontend * skip EditInspector if missing 'property_constraint_pid' in the manifest * improve unit tests for fetching lang codes * skip scrutinizers depending on fetcher if 'property_constraint_pid' is missing in the manifest * make sure the schema alignment panel is set up before rendering * fix preview bug * add getters of "instance of" and "subclass of" to the Manifest interface and use them in NewItemScrutinizer * fix hardcode for Wikidata in WbItemVariable * rename 'entity_prefix' to 'site_iri' and move it from 'manifest.wikibase.properties' to 'manifest.wikibase' * include oauth configurations in the manifest & support logging in with owner-only consumer for Wikibases with the OAuth extension * correct schema fallback logic * select default wikibase according to the saved schema * include maxlag in the manifest * [backend] move maxlag setting from preferences to request parameter * support setting maxlag when uploading edits * rename "Manage Wikibase" to "Select Wikibase instance" and localize it * fix manifest updating bug * include EditGroups in the manifest * add the reconciliation service from the manifest to standard services if it's not present yet when adding a new manifest * update according to review feedback 1. use inherited color variable 2. rename 'gridwroks' to 'openrefine' 3. remove unnecessary 'async: true' 4. add 'format: url' validation to urls to the schema * rename 'wikibasePrefix' to 'siteIri'
154 lines
4.1 KiB
JavaScript
154 lines
4.1 KiB
JavaScript
// Load the localization file
|
|
var dictionary = {};
|
|
$.ajax({
|
|
url: "command/core/load-language?",
|
|
type: "POST",
|
|
async: false,
|
|
data: {
|
|
module: "wikidata",
|
|
// lang : lang
|
|
},
|
|
success: function (data) {
|
|
dictionary = data['dictionary'];
|
|
lang = data['lang'];
|
|
}
|
|
});
|
|
$.i18n().load(dictionary, lang);
|
|
|
|
|
|
ExporterManager.MenuItems.push({});
|
|
ExporterManager.MenuItems.push({
|
|
id: "performWikibaseEdits",
|
|
label: $.i18n('wikibase-extension/wikibase-edits'),
|
|
click: function () {
|
|
PerformEditsDialog.checkAndLaunch();
|
|
}
|
|
});
|
|
ExporterManager.MenuItems.push({
|
|
id: "exportQuickStatements",
|
|
label: $.i18n('wikibase-extension/qs-file'),
|
|
click: function () {
|
|
WikibaseExporterMenuBar.checkSchemaAndExport("quickstatements");
|
|
}
|
|
});
|
|
ExporterManager.MenuItems.push({
|
|
id: "exportWikibaseSchema",
|
|
label: $.i18n('wikibase-extension/wikibase-schema'),
|
|
click: function () {
|
|
WikibaseExporterMenuBar.checkSchemaAndExport("wikibase-schema");
|
|
}
|
|
});
|
|
|
|
WikibaseExporterMenuBar = {};
|
|
|
|
WikibaseExporterMenuBar.exportTo = function (format) {
|
|
var targetUrl = null;
|
|
if (format === "quickstatements") {
|
|
targetUrl = "statements.txt";
|
|
} else {
|
|
targetUrl = "schema.json";
|
|
}
|
|
var form = document.createElement("form");
|
|
$(form).css("display", "none")
|
|
.attr("method", "post")
|
|
.attr("action", "command/core/export-rows/" + targetUrl)
|
|
.attr("target", "openrefine-export-" + format);
|
|
$('<input />')
|
|
.attr("name", "engine")
|
|
.val(JSON.stringify(ui.browsingEngine.getJSON()))
|
|
.appendTo(form);
|
|
$('<input />')
|
|
.attr("name", "project")
|
|
.val(theProject.id)
|
|
.appendTo(form);
|
|
$('<input />')
|
|
.attr("name", "format")
|
|
.val(format)
|
|
.appendTo(form);
|
|
|
|
document.body.appendChild(form);
|
|
|
|
window.open("about:blank", "openrefine-export");
|
|
form.submit();
|
|
|
|
document.body.removeChild(form);
|
|
};
|
|
|
|
WikibaseExporterMenuBar.checkSchemaAndExport = function (format) {
|
|
var onSaved = function (callback) {
|
|
WikibaseExporterMenuBar.exportTo(format);
|
|
};
|
|
if (!SchemaAlignment.isSetUp()) {
|
|
SchemaAlignment.launch(null);
|
|
} else if (SchemaAlignment._hasUnsavedChanges) {
|
|
SchemaAlignment._save(onSaved);
|
|
} else {
|
|
onSaved();
|
|
}
|
|
};
|
|
|
|
//extend the column header menu
|
|
$(function () {
|
|
|
|
ExtensionBar.MenuItems.push(
|
|
{
|
|
"id": "reconcile",
|
|
"label": $.i18n('wikibase-extension/menu-label'),
|
|
"submenu": [
|
|
{
|
|
id: "wikidata/select-instance",
|
|
label: $.i18n('wikibase-extension/select-wikibase-instance'),
|
|
click: function () {
|
|
WikibaseDialog.launch()
|
|
}
|
|
},
|
|
{
|
|
id: "wikidata/edit-schema",
|
|
label: $.i18n('wikibase-extension/edit-wikibase-schema'),
|
|
click: function () {
|
|
SchemaAlignment.launch(false);
|
|
}
|
|
},
|
|
{
|
|
id: "wikidata/manage-account",
|
|
label: $.i18n('wikibase-extension/manage-wikibase-account'),
|
|
click: function () {
|
|
ManageAccountDialog.checkAndLaunch();
|
|
}
|
|
},
|
|
{},
|
|
{
|
|
id: "wikidata/import-schema",
|
|
label: $.i18n('wikibase-extension/import-wikibase-schema'),
|
|
click: function () {
|
|
ImportSchemaDialog.launch();
|
|
}
|
|
},
|
|
{
|
|
id: "wikidata/export-schema",
|
|
label: $.i18n('wikibase-extension/export-schema'),
|
|
click: function () {
|
|
WikibaseExporterMenuBar.checkSchemaAndExport("wikibase-schema");
|
|
}
|
|
},
|
|
{},
|
|
{
|
|
id: "wikidata/perform-edits",
|
|
label: $.i18n('wikibase-extension/perform-edits-on-wikibase'),
|
|
click: function () {
|
|
PerformEditsDialog.checkAndLaunch();
|
|
}
|
|
},
|
|
{
|
|
id: "wikidata/export-qs",
|
|
label: $.i18n('wikibase-extension/export-to-qs'),
|
|
click: function () {
|
|
WikibaseExporterMenuBar.checkSchemaAndExport("quickstatements");
|
|
}
|
|
}
|
|
]
|
|
}
|
|
);
|
|
});
|
|
|