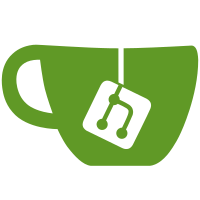
Closes #1640. All Wikibase-dependent parameters, which were previously hard-coded for Wikidata, are now described in a JSON manifest. The manifest is currently constructed by hand, but, in the future, will hopefully be published by each Wikibase instance at a standard location. * setup the manifest framework * add dependency mechanism to scrutinizers & update tests * add json creators to constraint entities * adapt the backend (units tests are to be updated) * remove the call to prepareDependencies() in the constructor * update code according to review feedback * update scrutinizers tests * fix typo & update ConstraintsV1 * log if a scrutinizer is skipped * update versioning handling in the backend * correct the order of "actual" and "expected" for assertEquals method * use regex to check manifest versions * 1. add wikibase-manager.js, wikibase-dialog.js, etc. 2. move dialog/schema-alignment-dialog.js -> schema-alignment.js 3. remove unused schema-alignment-dialog.html 4. change most mentions of "Wikidata" to "Wikibase" * support saving cookies for different Wikibases & fix LoginCommandTest * fix schema related tests * removed unused WikibaseCredentials * include MediaWiki API endpoint in the schema * fetch language codes for different Wikibases * fix lgtm-bot alerts * keep a connection map (MediaWiki API endpoint => Connection) in ConnectionManager * simplify the constraint configurations of the manifest and remove lots of unnecessary code. * add slash to the end of mediawiki.root * add manifest schema and use ajv to validate the manifest * remove JSONP support (Wikibase manifest host should support CORS) * save manifests on manifest update * add unit tests for Manifest * include the exception in logger.error() method to make it easier to debug * include the message of ManifestException when calling respondError * test multiple connections * test no manifest & test invalid manifest * adapt manage-account-dialog.js to support multiple Wikibase connections * update instance/subclass of related translations * beautify import-schema-dialog.html * use "${lang}" variable in the reconciliation service endpoint of the manifest * adapt schema-alignment.js after introducing "${lang}" variable in the reconciliation service endpoint * use WikibaseManager.getSelectedWikibaseApi() in SchemaAlignment._getPropertyType * replace more mentions of "Wikidata" to "Wikibase" * use WikibaseManager.getSelectedWikibaseApi() in previewrenderer.js * support fetching language codes of different Wikibases in the frontend * skip EditInspector if missing 'property_constraint_pid' in the manifest * improve unit tests for fetching lang codes * skip scrutinizers depending on fetcher if 'property_constraint_pid' is missing in the manifest * make sure the schema alignment panel is set up before rendering * fix preview bug * add getters of "instance of" and "subclass of" to the Manifest interface and use them in NewItemScrutinizer * fix hardcode for Wikidata in WbItemVariable * rename 'entity_prefix' to 'site_iri' and move it from 'manifest.wikibase.properties' to 'manifest.wikibase' * include oauth configurations in the manifest & support logging in with owner-only consumer for Wikibases with the OAuth extension * correct schema fallback logic * select default wikibase according to the saved schema * include maxlag in the manifest * [backend] move maxlag setting from preferences to request parameter * support setting maxlag when uploading edits * rename "Manage Wikibase" to "Select Wikibase instance" and localize it * fix manifest updating bug * include EditGroups in the manifest * add the reconciliation service from the manifest to standard services if it's not present yet when adding a new manifest * update according to review feedback 1. use inherited color variable 2. rename 'gridwroks' to 'openrefine' 3. remove unnecessary 'async: true' 4. add 'format: url' validation to urls to the schema * rename 'wikibasePrefix' to 'siteIri'
191 lines
5.6 KiB
JavaScript
191 lines
5.6 KiB
JavaScript
/**
|
|
* Manages Wikibase instances.
|
|
*/
|
|
const WikibaseManager = {
|
|
selected: "Wikidata",
|
|
wikibases: {
|
|
"Wikidata": WikidataManifestV1_0 // default one
|
|
}
|
|
};
|
|
|
|
WikibaseManager.getSelectedWikibase = function () {
|
|
return WikibaseManager.wikibases[WikibaseManager.selected];
|
|
};
|
|
|
|
WikibaseManager.getSelectedWikibaseRoot = function () {
|
|
return WikibaseManager.getSelectedWikibase().mediawiki.root;
|
|
};
|
|
|
|
WikibaseManager.getSelectedWikibaseMainPage = function () {
|
|
return WikibaseManager.getSelectedWikibase().mediawiki.main_page;
|
|
};
|
|
|
|
WikibaseManager.getSelectedWikibaseApi = function () {
|
|
return WikibaseManager.getSelectedWikibase().mediawiki.api;
|
|
};
|
|
|
|
WikibaseManager.getSelectedWikibaseName = function () {
|
|
return WikibaseManager.selected;
|
|
};
|
|
|
|
WikibaseManager.getSelectedWikibaseSiteIri = function () {
|
|
return WikibaseManager.getSelectedWikibase().wikibase.site_iri;
|
|
};
|
|
|
|
WikibaseManager.getSelectedWikibaseMaxlag = function() {
|
|
return WikibaseManager.getSelectedWikibase().wikibase.maxlag;
|
|
};
|
|
|
|
WikibaseManager.getSelectedWikibaseOAuth = function() {
|
|
return WikibaseManager.getSelectedWikibase().oauth;
|
|
};
|
|
|
|
WikibaseManager.getSelectedWikibaseEditGroupsURLSchema = function() {
|
|
let editgroups = WikibaseManager.getSelectedWikibase().editgroups;
|
|
return editgroups ? editgroups.url_schema : null;
|
|
};
|
|
|
|
/**
|
|
* Returns the default reconciliation service URL of the Wikibase,
|
|
* such as "https://wdreconcile.toolforge.org/${lang}/api".
|
|
*
|
|
* Notice that there is a "${lang}" variable in the URL, which should
|
|
* be replaced with the actual language code.
|
|
*/
|
|
WikibaseManager.getSelectedWikibaseReconEndpoint = function () {
|
|
return WikibaseManager.getSelectedWikibase().reconciliation.endpoint;
|
|
};
|
|
|
|
WikibaseManager.selectWikibase = function (wikibaseName) {
|
|
if (WikibaseManager.wikibases.hasOwnProperty(wikibaseName)) {
|
|
WikibaseManager.selected = wikibaseName;
|
|
}
|
|
};
|
|
|
|
WikibaseManager.getAllWikibases = function () {
|
|
return WikibaseManager.wikibases;
|
|
};
|
|
|
|
WikibaseManager.addWikibase = function (manifest) {
|
|
WikibaseManager.wikibases[manifest.mediawiki.name] = manifest;
|
|
WikibaseManager.saveWikibases();
|
|
};
|
|
|
|
WikibaseManager.removeWikibase = function (wikibaseName) {
|
|
delete WikibaseManager.wikibases[wikibaseName];
|
|
WikibaseManager.saveWikibases();
|
|
};
|
|
|
|
WikibaseManager.saveWikibases = function () {
|
|
let manifests = [];
|
|
for (let wikibaseName in WikibaseManager.wikibases) {
|
|
if (WikibaseManager.wikibases.hasOwnProperty(wikibaseName)) {
|
|
manifests.push(WikibaseManager.wikibases[wikibaseName])
|
|
}
|
|
}
|
|
|
|
Refine.wrapCSRF(function (token) {
|
|
$.ajax({
|
|
async: false,
|
|
type: "POST",
|
|
url: "command/core/set-preference?" + $.param({
|
|
name: "wikibase.manifests"
|
|
}),
|
|
data: {
|
|
"value": JSON.stringify(manifests),
|
|
csrf_token: token
|
|
},
|
|
dataType: "json"
|
|
});
|
|
});
|
|
};
|
|
|
|
WikibaseManager.loadWikibases = function (onDone) {
|
|
$.ajax({
|
|
url: "command/core/get-preference?" + $.param({
|
|
name: "wikibase.manifests"
|
|
}),
|
|
success: function (data) {
|
|
if (data.value && data.value !== "null" && data.value !== "[]") {
|
|
let manifests = JSON.parse(data.value);
|
|
manifests.forEach(function (manifest) {
|
|
if (manifest.custom && manifest.custom.url && manifest.custom.last_updated
|
|
&& ((Date.now() - new Date(manifest.custom.last_updated)) > 7 * 24 * 60 * 60 * 1000)) {
|
|
// If the manifest was fetched via URL and hasn't been updated for a week,
|
|
// fetch it again to keep track of the lasted version
|
|
WikibaseManager.fetchManifestFromURL(manifest.custom.url, function (newManifest) {
|
|
WikibaseManager.wikibases[newManifest.mediawiki.name] = newManifest;
|
|
WikibaseManager.saveWikibases();
|
|
}, function () {
|
|
// fall back to the current one if failed to fetch the latest one
|
|
WikibaseManager.wikibases[manifest.mediawiki.name] = manifest;
|
|
}, true)
|
|
} else {
|
|
WikibaseManager.wikibases[manifest.mediawiki.name] = manifest;
|
|
}
|
|
});
|
|
|
|
WikibaseManager.selected = WikibaseManager.selectDefaultWikibaseAccordingToSavedSchema();
|
|
|
|
if (onDone) {
|
|
onDone();
|
|
}
|
|
}
|
|
},
|
|
dataType: "json"
|
|
});
|
|
};
|
|
|
|
WikibaseManager.selectDefaultWikibaseAccordingToSavedSchema = function () {
|
|
let schema = theProject.overlayModels.wikibaseSchema || {};
|
|
if (!schema.siteIri) {
|
|
return "Wikidata";
|
|
}
|
|
|
|
for (let wikibaseName in WikibaseManager.wikibases) {
|
|
if (WikibaseManager.wikibases.hasOwnProperty(wikibaseName)) {
|
|
let wikibase = WikibaseManager.wikibases[wikibaseName];
|
|
if (schema.siteIri === wikibase.wikibase.site_iri) {
|
|
return wikibase.mediawiki.name;
|
|
}
|
|
}
|
|
}
|
|
|
|
return "Wikidata";
|
|
};
|
|
|
|
WikibaseManager.fetchManifestFromURL = function (manifestURL, onSuccess, onError, silent) {
|
|
let dismissBusy = function() {};
|
|
if (!silent) {
|
|
dismissBusy = DialogSystem.showBusy($.i18n("wikibase-management/contact-service") + "...");
|
|
}
|
|
|
|
let _onSuccess = function (data) {
|
|
// record custom information in the manifest
|
|
data.custom = {};
|
|
data.custom.url = manifestURL;
|
|
data.custom.last_updated = Date.now();
|
|
if (onSuccess) {
|
|
onSuccess(data);
|
|
}
|
|
};
|
|
|
|
// The manifest host must support CORS.
|
|
$.ajax(manifestURL, {
|
|
"dataType": "json",
|
|
"timeout": 5000
|
|
}).success(function (data) {
|
|
dismissBusy();
|
|
_onSuccess(data);
|
|
}).error(function (jqXHR, textStatus, errorThrown) {
|
|
dismissBusy();
|
|
if (!silent) {
|
|
alert($.i18n("wikibase-management/error-contact")+": " + textStatus + " : " + errorThrown + " - " + manifestURL);
|
|
}
|
|
if (onError) {
|
|
onError();
|
|
}
|
|
});
|
|
};
|
|
|