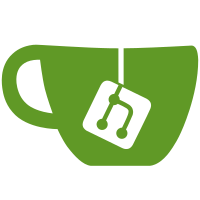
Target reached now checks if target is closer than firklift speed to avoid skipping the target location.Magazine can now be exported as graph.
104 lines
2.5 KiB
JavaScript
104 lines
2.5 KiB
JavaScript
let sections;
|
|
let roads;
|
|
let packageClaim;
|
|
let going = false;
|
|
let forklift;
|
|
|
|
// This runs once at start
|
|
function setup() {
|
|
createCanvas(500, 500).parent('canvas');
|
|
|
|
frameRate(30);
|
|
|
|
createMagazineLayout();
|
|
|
|
select('#button').mousePressed(deliver);
|
|
// Create a forklift instance
|
|
forklift = new Forklift(sections[0].x, sections[0].y);
|
|
console.log(magazineToGraph());
|
|
}
|
|
|
|
// This runs every frame
|
|
function draw() {
|
|
background(64);
|
|
drawMagazine();
|
|
forklift.draw();
|
|
if (going) {
|
|
if (forklift.targetReached()) {
|
|
going = false;
|
|
} else {
|
|
forklift.move();
|
|
}
|
|
}
|
|
}
|
|
|
|
function drawMagazine() {
|
|
noFill();
|
|
stroke(220);
|
|
strokeWeight(10);
|
|
// Draw all the roads in the magazine
|
|
for (let road of roads) {
|
|
line(
|
|
sections[road[0]].x,
|
|
sections[road[0]].y,
|
|
sections[road[1]].x,
|
|
sections[road[1]].y
|
|
);
|
|
}
|
|
noStroke();
|
|
textAlign(CENTER, CENTER);
|
|
// Draw all sections in the magazine
|
|
for (let section of Object.keys(sections)) {
|
|
if (section === 0) {
|
|
fill(80);
|
|
rect(sections[section].x - 15, sections[section].y, 30, 30);
|
|
} else {
|
|
fill(30);
|
|
ellipse(sections[section].x, sections[section].y, 30);
|
|
fill(255);
|
|
text(section, sections[section].x, sections[section].y);
|
|
}
|
|
}
|
|
}
|
|
|
|
function deliver() {
|
|
path = [2, 1, 5, 4];
|
|
forklift.setPath(path);
|
|
going = true;
|
|
}
|
|
|
|
function createMagazineLayout() {
|
|
unit = width / 5;
|
|
|
|
sections = {
|
|
0: { x: unit * 2, y: 0 },
|
|
1: createVector(unit, unit),
|
|
2: createVector(2 * unit, unit),
|
|
3: createVector(2 * unit, 2 * unit),
|
|
4: createVector(3 * unit, 2 * unit),
|
|
5: createVector(unit, 3 * unit),
|
|
6: createVector(2 * unit, 3 * unit)
|
|
};
|
|
roads = [[0, 2], [1, 2], [1, 5], [5, 4], [5, 6], [4, 3], [2, 3], [3, 6]];
|
|
}
|
|
|
|
function magazineToGraph() {
|
|
graph = {};
|
|
for (let key of Object.keys(sections)) {
|
|
graph[key] = {};
|
|
}
|
|
for (let road of roads) {
|
|
start = road[0];
|
|
end = road[1];
|
|
graph[start][end] = Math.sqrt(
|
|
Math.pow(sections[start].x - sections[end].x, 2) +
|
|
Math.pow(sections[start].y - sections[end].y, 2)
|
|
);
|
|
graph[end][start] = Math.sqrt(
|
|
Math.pow(sections[start].x - sections[end].x, 2) +
|
|
Math.pow(sections[start].y - sections[end].y, 2)
|
|
);
|
|
}
|
|
return graph;
|
|
}
|