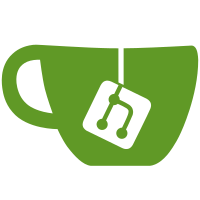
Bug where forklift couldnt get to certain nodes is removed by reimplementing vecdtor addition and substarction.
65 lines
1.6 KiB
Python
65 lines
1.6 KiB
Python
from django.shortcuts import render
|
|
from django.http import HttpResponse
|
|
from django.views.decorators.csrf import csrf_exempt
|
|
|
|
import json
|
|
import math
|
|
|
|
# Create your views here.
|
|
|
|
|
|
def index(request):
|
|
return HttpResponse('It lives!')
|
|
|
|
|
|
@csrf_exempt
|
|
def classify(request):
|
|
return HttpResponse(json.load(request))
|
|
|
|
|
|
@csrf_exempt
|
|
def shortestPath(request):
|
|
loaded_request = json.load(request)
|
|
|
|
graph = loaded_request["graph"]
|
|
graph = {int(k): v for k, v in graph.items()}
|
|
for node in graph:
|
|
graph[node] = {
|
|
int(k): v for k, v in graph[node].items()}
|
|
|
|
start_node = loaded_request["start_node"]
|
|
dest_node = loaded_request["dest_node"]
|
|
|
|
distance = {}
|
|
predecessor = {}
|
|
path = {}
|
|
unseen_nodes = graph.copy()
|
|
|
|
for node in graph:
|
|
distance[node] = math.inf
|
|
distance[start_node] = 0
|
|
|
|
while unseen_nodes:
|
|
min_node = None
|
|
for node in unseen_nodes:
|
|
if min_node is None:
|
|
min_node = node
|
|
elif distance[node] < distance[min_node]:
|
|
min_node = node
|
|
|
|
for childNode, weight in graph[min_node].items():
|
|
if distance[min_node] + weight < distance[childNode]:
|
|
distance[childNode] = distance[min_node] + weight
|
|
predecessor[childNode] = min_node
|
|
unseen_nodes.pop(min_node)
|
|
|
|
for node in graph:
|
|
current = node
|
|
p = [current]
|
|
while current != start_node:
|
|
p.append(predecessor[current])
|
|
current = predecessor[current]
|
|
path[node] = p[::-1]
|
|
|
|
return HttpResponse(path[dest_node][1:])
|