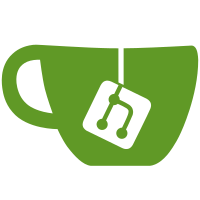
Add AutoMapper and INotifyPropertyChanged to enable cart quantity changes to be reflected
42 lines
1.0 KiB
C#
42 lines
1.0 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.ComponentModel;
|
|
using System.Linq;
|
|
using System.Text;
|
|
using System.Threading.Tasks;
|
|
|
|
namespace RMWPFUserInterface.Models
|
|
{
|
|
public class CartItemDisplayModel : INotifyPropertyChanged
|
|
{
|
|
public ProductDisplayModel Product { get; set; }
|
|
private int _quantityInCart;
|
|
|
|
public int QuantityInCart
|
|
{
|
|
get { return _quantityInCart; }
|
|
set
|
|
{
|
|
_quantityInCart = value;
|
|
CallPropertyChanges(nameof(QuantityInCart));
|
|
CallPropertyChanges(nameof(DisplayText));
|
|
}
|
|
}
|
|
|
|
public string DisplayText
|
|
{
|
|
get
|
|
{
|
|
return $"{Product.ProductName} ({QuantityInCart})";
|
|
}
|
|
}
|
|
|
|
public event PropertyChangedEventHandler PropertyChanged;
|
|
public void CallPropertyChanges(string propertyName)
|
|
{
|
|
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName));
|
|
}
|
|
}
|
|
}
|
|
|