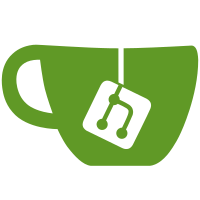
Connected the login form button to the Authentication endpoint (/token). Gets back bearer token or exception if failed
54 lines
1.7 KiB
C#
54 lines
1.7 KiB
C#
using RMWPFUserInterface.Models;
|
|
using System;
|
|
using System.Collections.Generic;
|
|
using System.Configuration;
|
|
using System.Linq;
|
|
using System.Net.Http;
|
|
using System.Net.Http.Headers;
|
|
using System.Text;
|
|
using System.Threading.Tasks;
|
|
|
|
namespace RMWPFUserInterface.Helpers
|
|
{
|
|
public class APIHelper : IAPIHelper
|
|
{
|
|
private HttpClient apiClient;
|
|
public APIHelper()
|
|
{
|
|
InitializeClient();
|
|
}
|
|
private void InitializeClient()
|
|
{
|
|
string api = ConfigurationManager.AppSettings["api"];
|
|
|
|
apiClient = new HttpClient();
|
|
apiClient.BaseAddress = new Uri(api);
|
|
apiClient.DefaultRequestHeaders.Accept.Clear();
|
|
apiClient.DefaultRequestHeaders.Accept.Add(new MediaTypeWithQualityHeaderValue("application/json"));
|
|
}
|
|
|
|
public async Task<AuthenticatedUser> Authenticate(string username, string password)
|
|
{
|
|
var data = new FormUrlEncodedContent(new[]
|
|
{
|
|
new KeyValuePair<string, string>("grant_type", "password"),
|
|
new KeyValuePair<string, string>("username", username),
|
|
new KeyValuePair<string, string>("password", password),
|
|
});
|
|
|
|
using (HttpResponseMessage response = await apiClient.PostAsync("/Token", data))
|
|
{
|
|
if (response.IsSuccessStatusCode)
|
|
{
|
|
var result = await response.Content.ReadAsAsync<AuthenticatedUser>();
|
|
return result;
|
|
}
|
|
else
|
|
{
|
|
throw new Exception(response.ReasonPhrase);
|
|
}
|
|
}
|
|
}
|
|
}
|
|
}
|