Compare commits
No commits in common. "master" and "raports" have entirely different histories.
5
.gitignore
vendored
@ -1,8 +1,5 @@
|
||||
VENV
|
||||
WENV
|
||||
env
|
||||
**/__pycache__
|
||||
.vscode
|
||||
*.swp
|
||||
linux_env
|
||||
moveset_data.json
|
||||
.vscode
|
||||
|
@ -1,26 +0,0 @@
|
||||
import pygame, random
|
||||
|
||||
from DataModels.Container import Container
|
||||
|
||||
from PIL import Image,ImageDraw
|
||||
from config import CELL_SIZE
|
||||
|
||||
class Cell( pygame.sprite.Sprite ):
|
||||
def __init__( self, x, y, max_rubbish, yellow = 0, green = 0, blue = 0, image_name = None):
|
||||
pygame.sprite.Sprite.__init__( self )
|
||||
self.image_name = image_name or type(self).__name__
|
||||
self.update_rect( x,y )
|
||||
self.container = Container( max_rubbish, yellow, green, blue )
|
||||
self.update_image()
|
||||
|
||||
def update_rect( self, x, y ):
|
||||
self.x, self.y = x,y
|
||||
self.rect = pygame.Rect( x * CELL_SIZE, y * CELL_SIZE, CELL_SIZE, CELL_SIZE )
|
||||
|
||||
def update_image( self ):
|
||||
image = Image.open("Resources/Images/Image_" + self.image_name + ".png" )
|
||||
draw = ImageDraw.Draw(image)
|
||||
draw.text( (5,5), str( self.container.status() ) )
|
||||
mode, size, data = image.mode, image.size, image.tobytes()
|
||||
|
||||
self.image = pygame.image.frombuffer( data, size, mode )
|
@ -1,41 +0,0 @@
|
||||
class Container():
|
||||
def __init__(self, max, y=0, g=0, b=0):
|
||||
self.yellow, self.green, self.blue = y, g, b
|
||||
self.max = max
|
||||
|
||||
def empty(self, trash_type=None, trash=None):
|
||||
if trash_type == None:
|
||||
self.yellow, self.green, self.blue = 0, 0, 0
|
||||
elif trash_type == "yellow":
|
||||
trash[0] += self.yellow
|
||||
self.yellow = 0
|
||||
elif trash_type == "green":
|
||||
trash[1] += self.green
|
||||
self.green = 0
|
||||
elif trash_type == "blue":
|
||||
trash[2] += self.blue
|
||||
self.blue = 0
|
||||
return trash
|
||||
|
||||
|
||||
def status(self):
|
||||
return [self.yellow, self.green, self.blue]
|
||||
|
||||
def is_full(self):
|
||||
if self.yellow>0 or self.green>0 or self.blue>0:
|
||||
return 1
|
||||
return 0
|
||||
|
||||
def add(self, trash):
|
||||
my_trash = [self.yellow, self.green, self.blue]
|
||||
leftovers = [0, 0, 0]
|
||||
for i in range(0, len(trash)):
|
||||
while(my_trash[i] < self.max and trash[i] > 0):
|
||||
my_trash[i] += 1
|
||||
trash[i] -= 1
|
||||
self.yellow, self.green, self.blue = my_trash
|
||||
result = [0, 0, 0]
|
||||
for i in range(0, len(trash)):
|
||||
result[i] = trash[i] - leftovers[i]
|
||||
|
||||
return result
|
@ -1,14 +0,0 @@
|
||||
import pygame
|
||||
from DataModels.Cell import Cell
|
||||
|
||||
class Dump( Cell ):
|
||||
def __init__( self, x, y, max_rubbish, dump_type, yellow = 0, green = 0, blue = 0 ):
|
||||
Cell.__init__( self, x, y, max_rubbish, yellow, green, blue, dump_type )
|
||||
self.dump_type = dump_type
|
||||
self.unvisited = True
|
||||
|
||||
def return_trash(self, collector):
|
||||
dump_type = self.dump_type.lower()[5:]
|
||||
self.container.yellow, self.container.green, self.container.blue = collector.container.empty(dump_type,
|
||||
[self.container.yellow, self.container.green, self.container.blue])
|
||||
self.update_image()
|
120
DataModels/GC.py
@ -1,120 +0,0 @@
|
||||
from DataModels.Cell import Cell
|
||||
from DataModels.Road import Road
|
||||
from DataModels.House import House
|
||||
from DataModels.Dump import Dump
|
||||
from config import GRID_WIDTH, GRID_HEIGHT, DELAY
|
||||
from utilities import movement, check_moves, save_moveset
|
||||
from Traversal.DFS import DFS
|
||||
from Traversal.BestFS import BestFS
|
||||
from Traversal.BFS import BFS
|
||||
import pygame
|
||||
|
||||
class GC(Cell):
|
||||
moves_made = 0
|
||||
def __init__(self, x, y, max_rubbish, yellow=0, green=0, blue=0):
|
||||
Cell.__init__(self, x, y, max_rubbish, yellow, green, blue)
|
||||
self.moves = []
|
||||
self.old_time = pygame.time.get_ticks()
|
||||
def move(self, direction, environment):
|
||||
self.x, self.y = movement(environment, self.x, self.y)[0][direction]
|
||||
self.update_rect(self.x, self.y)
|
||||
self.moves_made = self.moves_made + 1 #moves counter
|
||||
|
||||
print(check_moves(environment, self.x, self.y,direction))
|
||||
|
||||
def collect(self, enviromnent):
|
||||
x, y = [self.x, self.y]
|
||||
coordinates = [(x, y - 1), (x, y + 1), (x - 1, y), (x + 1, y)]
|
||||
for coordinate in coordinates:
|
||||
if coordinate[0]<0 or coordinate[1]<0:
|
||||
continue
|
||||
try:
|
||||
item = enviromnent[coordinate[0]][coordinate[1]]
|
||||
except:
|
||||
continue
|
||||
|
||||
if(type(item) == House or type(item) == Dump):
|
||||
item.return_trash(self)
|
||||
self.update_image()
|
||||
|
||||
def get_moves_count(self):
|
||||
return self.moves_made
|
||||
|
||||
def find_houses(self,enviromnent, house_count,dump_count, mode):
|
||||
x = self.x
|
||||
y = self.y
|
||||
result = []
|
||||
element_list=[]
|
||||
house_count_after_search=house_count
|
||||
for home in range(house_count):
|
||||
avalible_moves = check_moves(enviromnent, x,y)
|
||||
if mode == "DFS":
|
||||
house,[x,y],result = DFS(enviromnent,avalible_moves,[[x,y]],House)
|
||||
elif mode == "BFS":
|
||||
house,[x,y],result = BFS(enviromnent,avalible_moves,[[x,y]],House)
|
||||
result = result[1::]
|
||||
self.moves.extend(result)
|
||||
element_list.append(house)
|
||||
|
||||
for dump in range(dump_count):
|
||||
avalible_moves = check_moves(enviromnent, x,y)
|
||||
if mode == "DFS":
|
||||
dump,[x,y],result = DFS(enviromnent,avalible_moves,[[x,y]],Dump)
|
||||
elif mode == "BFS":
|
||||
dump,[x,y],result = BFS(enviromnent,avalible_moves,[[x,y]],Dump)
|
||||
self.moves.extend(result)
|
||||
element_list.append(dump)
|
||||
for x in element_list:
|
||||
x.unvisited = True
|
||||
self.moves.reverse()
|
||||
save_moveset(self.moves)
|
||||
|
||||
|
||||
def find_houses_BestFS(self, environment):
|
||||
x = self.x
|
||||
y = self.y
|
||||
result = [[x,y]]
|
||||
|
||||
houses_list = []
|
||||
dump_list = []
|
||||
a = 0
|
||||
for row in environment:
|
||||
b = 0
|
||||
for col in row:
|
||||
if (type(col) is House):
|
||||
houses_list.append([col,[a,b]])
|
||||
if (type(col) is Dump):
|
||||
dump_list.append([col,[a,b]])
|
||||
b += 1
|
||||
a += 1
|
||||
|
||||
x, y = self.x, self.y
|
||||
|
||||
for i in range(len(houses_list)):
|
||||
available_movement = check_moves(environment, x, y)
|
||||
output = BestFS(environment, available_movement, [[x,y]], houses_list)
|
||||
if(output != None):
|
||||
[x,y],result,houses_list = output[0], output[1], output[2]
|
||||
self.moves.extend(result[1:])
|
||||
for i in range(len(dump_list)):
|
||||
available_movement = check_moves(environment, x, y)
|
||||
output = BestFS(environment, available_movement, [[x,y]], dump_list)
|
||||
if(output != None):
|
||||
[x,y],result,dump_list = output[0], output[1], output[2]
|
||||
self.moves.extend(result[1:])
|
||||
self.moves.reverse()
|
||||
save_moveset(self.moves)
|
||||
|
||||
|
||||
def make_actions_from_list(self,environment):
|
||||
now = pygame.time.get_ticks()
|
||||
if len(self.moves)==0 or now - self.old_time <= DELAY:
|
||||
return
|
||||
self.old_time = pygame.time.get_ticks()
|
||||
if self.moves[-1] == "pick_garbage":
|
||||
self.collect(environment)
|
||||
self.moves.pop()
|
||||
return
|
||||
self.x, self.y = self.moves.pop()
|
||||
self.moves_made = self.moves_made + 1 #moves counter
|
||||
self.update_rect(self.x,self.y)
|
@ -1,8 +0,0 @@
|
||||
import pygame
|
||||
from config import CELL_SIZE
|
||||
|
||||
class Grass( pygame.sprite.Sprite ):
|
||||
def __init__( self, x, y ):
|
||||
pygame.sprite.Sprite.__init__( self )
|
||||
self.rect = pygame.Rect( x * CELL_SIZE, y * CELL_SIZE, CELL_SIZE, CELL_SIZE )
|
||||
self.image = pygame.image.load("Resources/Images/Image_Grass.png")
|
@ -1,12 +0,0 @@
|
||||
from DataModels.Cell import Cell
|
||||
|
||||
|
||||
class House(Cell):
|
||||
def __init__(self, x, y, max_rubbish, yellow=0, green=0, blue=0):
|
||||
Cell.__init__(self, x, y, max_rubbish, yellow, green, blue)
|
||||
self.unvisited = True
|
||||
|
||||
def return_trash(self, collector):
|
||||
self.container.yellow, self.container.green, self.container.blue = collector.container.add(
|
||||
[self.container.yellow, self.container.green, self.container.blue])
|
||||
self.update_image()
|
@ -1,8 +0,0 @@
|
||||
import pygame
|
||||
from config import CELL_SIZE
|
||||
|
||||
class Road( pygame.sprite.Sprite ):
|
||||
def __init__( self, x, y ):
|
||||
pygame.sprite.Sprite.__init__( self )
|
||||
self.rect = pygame.Rect( x * CELL_SIZE, y * CELL_SIZE, CELL_SIZE, CELL_SIZE )
|
||||
self.image = pygame.image.load("Resources/Images/Image_Road.png")
|
13
Makefile
Normal file
@ -0,0 +1,13 @@
|
||||
.PHONY: init install start
|
||||
|
||||
init-linux:
|
||||
python3 -m venv env
|
||||
|
||||
install:
|
||||
env/bin/pip3 install -r requirements.txt
|
||||
|
||||
start:
|
||||
env/bin/python3 ./game.py --home-count=5
|
||||
|
||||
|
||||
|
153
MapGenerator.py
@ -1,153 +0,0 @@
|
||||
import random, datetime, itertools
|
||||
|
||||
def GenerateMap():
|
||||
#generate random empty map
|
||||
width = random.randint(5,15) #up to 15
|
||||
height = random.randint(5,10) #up to 10
|
||||
grid = []
|
||||
|
||||
row = []
|
||||
for i in range(width):
|
||||
row.append('E')
|
||||
for i in range(height):
|
||||
grid.append(row.copy())
|
||||
|
||||
#define number of roads for each axis
|
||||
x_roads_count = random.randint(2, max(width//3,2))
|
||||
y_roads_count = random.randint(2, max(height//3,2))
|
||||
|
||||
#select coords of roads for x
|
||||
x_roads_coordinates = [] #output coords
|
||||
possible_coordiantes = [i for i in range(width)] #coords to choose from
|
||||
|
||||
for i in range(x_roads_count):
|
||||
coordinate = random.choice(possible_coordiantes)
|
||||
road_area = [coordinate-1, coordinate, coordinate+1]
|
||||
possible_coordiantes = [i for i in possible_coordiantes if i not in road_area] #removes road and surrounding coords (total 3 coords) from possible coords
|
||||
x_roads_coordinates.append(coordinate)
|
||||
|
||||
#select coords of roads for y
|
||||
y_roads_coordinates = [] #output coords
|
||||
possible_coordiantes = [i for i in range(height)] #coords to choose from
|
||||
|
||||
for i in range(y_roads_count):
|
||||
coordinate = random.choice(possible_coordiantes)
|
||||
road_area = [coordinate-1, coordinate, coordinate+1]
|
||||
possible_coordiantes = [i for i in possible_coordiantes if i not in road_area] #removes road and surrounding coords (total 3 coords) from possible coords
|
||||
y_roads_coordinates.append(coordinate)
|
||||
|
||||
#create list of road coordinates
|
||||
roads = []
|
||||
for x in x_roads_coordinates:
|
||||
for y in range(height):
|
||||
roads.append([x,y])
|
||||
|
||||
for y in y_roads_coordinates:
|
||||
for x in range(width):
|
||||
roads.append([x,y])
|
||||
|
||||
"""AH SHIT HERE WE GO AGAIN"""
|
||||
#create list of path coords that can become new intersections by removing intersections and 8 adjacent tiles from roads
|
||||
intersections_area = []
|
||||
for x in x_roads_coordinates:
|
||||
for y in y_roads_coordinates:
|
||||
intersection_area = []
|
||||
for i in range (-1,2,1):
|
||||
for j in range (-1,2,1):
|
||||
intersection_area.append([x+i,y+j])
|
||||
intersections_area.extend(intersection_area)
|
||||
|
||||
possible_roads_to_modify = [i for i in roads if i not in intersections_area]
|
||||
roads_to_modify = []
|
||||
for i in range(1,len(possible_roads_to_modify)//3):
|
||||
choice = random.choice(possible_roads_to_modify)
|
||||
possible_roads_to_modify.remove(choice)
|
||||
roads_to_modify.append(choice)
|
||||
|
||||
"""CREATION TIME"""
|
||||
#perform modification based on road
|
||||
for r in roads_to_modify:
|
||||
#select possible directions for modification
|
||||
x, y = r
|
||||
direction = random.choice([1,-1])
|
||||
if([x+1, y] in roads or [x-1, y] in roads):
|
||||
#modify y, as there is road on x coordinates
|
||||
route = []
|
||||
current_tile = [x,y+direction]
|
||||
while(True):
|
||||
if(current_tile[1]<0 or current_tile[1]>=height or current_tile in roads):
|
||||
break
|
||||
else:
|
||||
route.append(current_tile)
|
||||
current_tile = [current_tile[0],current_tile[1]+direction]
|
||||
else:
|
||||
#modify x, as there is road on y coordinates
|
||||
route = []
|
||||
current_tile = [x+direction,y]
|
||||
while(True):
|
||||
if(current_tile[0]<0 or current_tile[0]>=width or current_tile in roads):
|
||||
break
|
||||
else:
|
||||
route.append(current_tile)
|
||||
current_tile = [current_tile[0]+direction,current_tile[1]]
|
||||
|
||||
#choose if the route should be complete or not
|
||||
if(len(route)>1):
|
||||
if(random.randint(1,100)<=65): #40% chance for route not to be full length
|
||||
#route.reverse()
|
||||
route_len = random.randint(1,len(route)-1)
|
||||
route = route[0:route_len]
|
||||
|
||||
#add new route to roads
|
||||
roads.extend(route)
|
||||
|
||||
#insert roads into the grid
|
||||
for coord in roads:
|
||||
grid[coord[1]][coord[0]] = "R"
|
||||
|
||||
"""OBJECTS BE HERE"""
|
||||
#Select area that possibly could hold objects
|
||||
objects_area = []
|
||||
for r in roads:
|
||||
objects_area.extend(([r[0]+1,r[1]],[r[0]-1,r[1]],[r[0],r[1]+1],[r[0],r[1]-1]))
|
||||
objects_area = [c for c in objects_area if c not in roads] #remove coords that contain roads
|
||||
objects_area.sort()
|
||||
objects_area = [objects_area[i] for i in range(len(objects_area)) if i == 0 or objects_area[i] != objects_area[i-1]] #remove duplicates
|
||||
|
||||
houses_area = [i.copy() for i in objects_area]
|
||||
for o in objects_area:
|
||||
if(o[0] < 0 or o[1] < 0 or o[0] >= width or o[1] >= height):
|
||||
houses_area.remove(o) #remove coords outside borders
|
||||
|
||||
#place dumps
|
||||
dumps_to_place = ["B","Y","G"]
|
||||
while(len(dumps_to_place) > 0):
|
||||
dump_coords = random.choice(houses_area)
|
||||
houses_area.remove(dump_coords)
|
||||
grid[dump_coords[1]][dump_coords[0]] = dumps_to_place[0]
|
||||
dumps_to_place.remove(dumps_to_place[0])
|
||||
|
||||
|
||||
#leave random coordinates
|
||||
houses_to_leave_count = len(houses_area)//4
|
||||
while(len(houses_area) > houses_to_leave_count):
|
||||
houses_area.remove(random.choice(houses_area))
|
||||
|
||||
#insert houses into the grid
|
||||
for coord in houses_area:
|
||||
grid[coord[1]][coord[0]] = "H"
|
||||
|
||||
#Select position for GC
|
||||
GC_position = random.choice(roads)
|
||||
|
||||
#Save map to file
|
||||
name = ".\\Resources\\Maps\\map"+str(datetime.datetime.now().strftime("%Y%m%d%H%M%S"))+"_auto.txt"
|
||||
map_file = open(name, "w+")
|
||||
map_file.write(str(width)+" "+str(height)+"\n")
|
||||
map_file.write(str(GC_position[0])+" "+str(GC_position[1])+"\n")
|
||||
for row in grid:
|
||||
map_file.write(" ".join(row)+"\n")
|
||||
map_file.close()
|
||||
print(name)
|
||||
|
||||
return(name)
|
@ -1,6 +1,6 @@
|
||||
# Smieciarka WMI
|
||||
|
||||
## Lekkie notateczki
|
||||
## Lekki notateczki
|
||||
|
||||
```yaml
|
||||
Modele:
|
||||
|
@ -1,71 +0,0 @@
|
||||
# Sztuczna inteligencja 2019 - Raport 2
|
||||
|
||||
**Czas trwania opisywanych prac:** 06.03.2019 - 26.03.2019
|
||||
|
||||
**Członkowie zespołu:** Anna Nowak, Magdalena Wilczyńska, Konrad Pierzyński, Michał Starski
|
||||
|
||||
**Wybrany temat:** Inteligentna śmieciarka
|
||||
|
||||
**Link do repozytorium projektu:** https://git.wmi.amu.edu.pl/s440556/SZI2019SmieciarzWmi
|
||||
|
||||
## Pierwszy algorytm przeszukiwania mapy - DFS
|
||||
|
||||
#### Implementacja
|
||||
|
||||
Pierwszym podejściem naszej grupy do rozwiązania problemu była implementacja
|
||||
algorytmu przeszukiwania drzewa w głąb - DFS (Wersja rekurencyjna).
|
||||
Aby zaimplementować ten algorytm, niezbędne było przygotowanie dla niego kilku
|
||||
struktur pomocniczych dzięki którym będziemy mogli jasno zdefiniować warunki stopu i uzyskać satysfakcjonujące nas rozwiązanie.
|
||||
|
||||
Do użytych struktur należą:
|
||||
|
||||
- **Lista dwuwymiarowa przedstawiająca mapę w formie siatki po której można łatwo iterować** - Jeden stan takiej listy traktowaliśmy jako wierzchołek grafu
|
||||
- **Lista możliwych ruchów do wykonania przez agenta przy konkretnym stanie mapy**
|
||||
- **Licznik głębokości na którą zszedł algorytm** - Zapobiega zajściu za głęboko w przypadku braku rozwiązania
|
||||
|
||||
**Przebieg algorytmu**:
|
||||
1. Sprawdź czy w pobliżu śmieciarki znajduje się nieopróżnioony domek
|
||||
2. Jeżeli możliwa jest jakaś akcja (zebranie/oddanie smieci) wykonaj ją
|
||||
- Jeżeli akcja została wykonana, zakończ algorytm
|
||||
- Jeżeli nie, sprawdź czy głębokość przekroczyła 30
|
||||
1. Jeżeli tak, zakończ algorytm informacją o braku rozwiązania
|
||||
2. Jeżeli nie, kontynuuj algorytm
|
||||
3. Dla każdego możliwego kierunku wykonaj algorytm od punktu 1
|
||||
|
||||
Rozwiązanie następuje wtedy, gdy domek zostaje opróżniony. Algorytm zostaje wywołany tyle samo razy, ile jest domków. Agent nie zna położenia domków na mapie podczas działania algorytmu.
|
||||
|
||||
#### Obserwacje
|
||||
|
||||
Przede wszystkim, algorytm przeszukiwania w głąb działa dla tego problemu
|
||||
**zdecydowanie wolniej niż powinien**, przy jego działaniu łatwo wchodzić w głąb złej ścieżki, która nie doprowadzi nas do rozwiązania. Co prawda przy małej mapie algorytm radzi sobie z problemem, jednak z każdą kolejną większą
|
||||
jest co raz gorzej.
|
||||
|
||||
Problem można pokazać na przykładzie:
|
||||
|
||||
Załóżmy, że hipotetyczne drzewo T wygląda w taki sposób:
|
||||
|
||||
```
|
||||
a -- e
|
||||
|
|
||||
b
|
||||
|
|
||||
c
|
||||
|
|
||||
d
|
||||
.
|
||||
. - 100 wierzchołków
|
||||
.
|
||||
x
|
||||
```
|
||||
|
||||
Naszym rozwiązaniem będzie doprowadzenie agenta z wierzchołka startowego **a** do wierzchołka końcowego **b**. DFS odwiedzi najpierw wierzchołki po kolei od a aż do x i dopiero później przyjdzie do e. Naturalnie można stwierdzić, że to nie jest sposób którego szukamy. Oczywiście 100 wierzchołków to mała liczba dla komputera, ale co jak będzie ich 1000, 10 000, lub 1 000 000 ?
|
||||
|
||||
#### Podsumowanie
|
||||
|
||||
Szukanie w głąb miałoby sens w innych przypadkach, na przykład gdybyśmy chcieli znaleźć możliwe wyniki w grze gdzie każda akcja pociąga za sobą kolejną, tworząc dość głęboki graf.
|
||||
|
||||
Do szukania ścieżki po której ma poruszać się agent lepiej byłoby jednak przeszukiwać graf wszerz.
|
||||
|
||||
#### Uruchamianie
|
||||
|
||||
Aby uruchomić DFS na mapie Śmieciarza WMI, należy kliknąć **0**.
|
@ -1,64 +0,0 @@
|
||||
# Sztuczna inteligencja 2019 - Raport 3
|
||||
|
||||
**Czas trwania opisywanych prac:** 03.04.2019 - 14.04.2019
|
||||
|
||||
**Członkowie zespołu:** Anna Nowak, Magdalena Wilczyńska, Konrad Pierzyński, Michał Starski
|
||||
|
||||
**Wybrany temat:** Inteligentna śmieciarka
|
||||
|
||||
|
||||
**Link do repozytorium projektu:** https://git.wmi.amu.edu.pl/s440556/SZI2019SmieciarzWmi
|
||||
|
||||
## Planowanie ruchu - Algorytmy BFS i Best-first search
|
||||
|
||||
#### Implementacja
|
||||
|
||||
##### BFS (Iteracyjnie)
|
||||
Algorytm przeszukiwania drzewa w głąb.
|
||||
|
||||
Algorytm jest niepoinformowany.
|
||||
|
||||
W przypadku BFS użyte struktury pozostają w gruncie rzeczy te same (z tym, że tym razem zamiast stosu do przechowywania stanu używamy kolejki), zmienia się tylko kolejność wykonywanych instrukcji:
|
||||
|
||||
|
||||
**Przebieg algorytmu**:
|
||||
|
||||
- Dodaj do kolejki pierwszy krok
|
||||
- Dopóki kolejka nie jest pusta:
|
||||
1. Zdejmij z kolejki następny nieodwiedzony wierzchołek grafu
|
||||
2. Jeżeli możliwa jest jakaś akcja (zebranie/oddanie smieci) wykonaj ją
|
||||
3. Sprawdź wszystkie sąsiednie wierzchołki wybranego wierzchołka, które jeszcze nie zostały odwiedzone
|
||||
|
||||
##### Best-first search
|
||||
Agent idzie w kierunku celu do którego jest najbliżej w linii prostej w danym momencie.
|
||||
|
||||
Algorytm jest poinformowany.
|
||||
|
||||
**Przebieg algorytmu**
|
||||
|
||||
1. Ustaw pozycję początkową
|
||||
2. Znajdź obiekt w znajdujący się najbliżej w linii prostej
|
||||
3. Jeżeli odległość od obiektu wynosi 1, wykonaj interakcję i usuń obiekt z listy celów, zwróć ścieżkę do obiektu
|
||||
4. Jeżeli przekroczono limit rekursji lub nie można wykonać kroku zakończ
|
||||
5. Na podstawie pozycji nowoobranego celu wybierz preferowane oraz niechciane kierunki poruszania się
|
||||
6. Posortuj dozwolone ruchy zgodnie z preferencjami
|
||||
7. Dla każdego kierunku na liście przeszukuj dostępne ścieżki dopóki jakakolwiek nie zostanie znaleziona
|
||||
|
||||
|
||||
-----------
|
||||
Ponadto, dołożyliśmy śmieciarzowi możliwość oddawania śmieci na wysypisko w ten sposób kompletując założenia planszy.
|
||||
|
||||
#### Obserwacje
|
||||
W porównaniu do poprzednio zaimplementowanego DFS oba algorytmy sprawują się zdecydowanie szybciej. Przez to, że szukanie drogi nie odbywa się w głąb, agent nie traci czasu na przeszukiwanie wierzchołków z góry skazanych na porażkę. Poniżej przedstawiamy tabelę mierzącą liczbę kroków, która była potrzebna do wykonania przez agenta przy użyciu DFS, BFS i Best-first search na 5 przygotowanych do testów mapach:
|
||||
|
||||
| Algorytm / Kroki | Mapa 1 | Mapa 2 | Mapa 3 | Mapa 4 | Mapa 5 |
|
||||
| --- | --- | --- | --- | --- | --- |
|
||||
| DFS | 134 | 45 | 67 | 191 | 12 |
|
||||
| BFS | 62 | 23 | 57 | 101 | 12 |
|
||||
| Best-first | 55 | 20 | 58 | 99 | 12 |
|
||||
|
||||
Po wykonaniu testów możemy stwierdzić, że najlepszym z tych 3 algorytmów okazał się Best-first search. Warto jednak zauważyć, że różnica kroków jest mała.
|
||||
|
||||
Co widać bez jakichkolwiek wątpliwości DFS okazał się najgorszy (zgodnie z uzasadnieniem znajdującym się w raporcie nr. 2). Liczba kroków jest prawie dwukrotnie większa od tej w konkurujących algorytmach.
|
||||
|
||||
(Mapa 5 posiadała tylko jedną możliwe przejście posiadające 12 kroków ten wynik można pominąć.)
|
@ -1,89 +0,0 @@
|
||||
---
|
||||
|
||||
# SVM raport
|
||||
|
||||
##### Konrad Pierzyński
|
||||
|
||||
###### Śmieciarz
|
||||
|
||||
12.06.2019
|
||||
|
||||
---
|
||||
|
||||
**SVM** - **S**upport-**V**ector **M**achine - zestaw metod uczenia stosowanych głównie do klasyfikacji, której nauka ma na celu wyznaczenie płaszczyzn rozdzielających dane wejściowe na klasy.
|
||||
|
||||
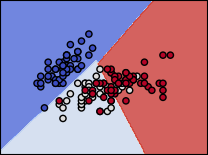
|
||||
|
||||
---
|
||||
|
||||
### Przygotowanie danych
|
||||
|
||||
Dane uczące zostały wygenerowane w następujący sposób:
|
||||
|
||||
+ Program generuje losową mapę o określonych wymiarach
|
||||
|
||||
+ Uruchamiany jest jeden z algorytmów (*BestFirstSearch*), który generuje listę ruchów.
|
||||
|
||||
+ Do zestawu uczącego dopisywana jest para składająca się na ruch i otoczenie gracza.
|
||||
|
||||
- Ruch odpowiada kierunkom: góra, prawo, dół, lewo i akcji zebrania/oddania śmieci - odpowienio liczbowo 1, 2, 3, 4, 99
|
||||
|
||||
- Otocznie to tablica dwuwymiarowa 7x7, gdzie element środkowy to pozycja gracza. Tablica ta następnie spłaszczana jest do tablicy jednowymiarowej
|
||||
|
||||
- Każdy 'domek', na którym została wykonana już akcja zebrania i jest opróżniony, widoczny jest na mapie tak samo jak element otoczenia, z którym gracz nie może wejść w żadną interakcję (stanąć, zebrać)
|
||||
|
||||
- Jeśli siatka 7x7 wykracza swoim zakresem za mapę, siatka uzupełniana jest przez trawę, czyli obiekt, z którym gracz nie wchodzi w interakcję
|
||||
|
||||
+ Po przejściu całej mapy algorytmem i zebraniu danych proces jest powtarzany tak długo, by zgromadzić około tysiąc rozwiązanych map
|
||||
|
||||
Pojedynczy zestaw danych jest zapisywany jako json postaci:
|
||||
|
||||
```json
|
||||
{
|
||||
"maps": [
|
||||
[Int, Int, ...],
|
||||
[Int, Int, ...],
|
||||
...
|
||||
],
|
||||
"moves":
|
||||
[
|
||||
Int, Int, ...
|
||||
]
|
||||
}
|
||||
```
|
||||
|
||||
I dopisywany do głównej struktury:
|
||||
|
||||
```json
|
||||
{
|
||||
"moveset": [
|
||||
Zestaw, Zestaw, ...
|
||||
]
|
||||
}
|
||||
```
|
||||
|
||||
---
|
||||
|
||||
### Uczenie
|
||||
|
||||
Do przeprowadzenia procesu uczenia dane uczące zostały podzielone na dwie listy:
|
||||
|
||||
- Pierwsza lista X zawiera wszystkie mapy częściowe (otoczenia)
|
||||
|
||||
```X = [ [Int, Int, ...], [Int, Int, ...], ... ]```
|
||||
|
||||
- Druga lista y zawiera odpowiadające mapom ruchy (1,2,3,4,99), które wykonał algorytm (*BestFirstSearch*) na danych otoczeniach.
|
||||
|
||||
```y = [ Int, Int, ... ]```
|
||||
|
||||
Wyżej wymienione dwie listy zostały podane jako argument metodzie ```fit(X,y)```, która odpowiada za uczenie się SVM. Natomiast utworzenie samego obiektu polega na zaimportowaniu biblioteki *scikit-learn*:
|
||||
```from sklearn import svm```
|
||||
a następnie już same utworzenia obiektu svm:
|
||||
```clf = svm.SVC(gamma='scale')```
|
||||
Wyuczony obiekt jest zapisywany do pliku, dzięki modułowi ```pickle```, aby nie przeprowadzać procesu uczenia za każdym uruchomieniem programu.
|
||||
|
||||
---
|
||||
|
||||
### Wykonywanie ruchów
|
||||
|
||||
Do przewidywania ruchów wystarczy użyć metody ```predict([ [otoczenie] ])``` , które przyjmuje mapę częściową, a jej wynik jest akcją, którą powinien wykonać gracz. Wynik metody przekazywany jest graczowi, który wykonuje ruch.
|
@ -1,92 +0,0 @@
|
||||
# Sztuczna Inteligencja 2019 - Raport Indywidualny
|
||||
|
||||
**Czas trwania opisywanych prac:** 09.05.2019 - 11.06.2019
|
||||
|
||||
**Autor:** Michał Starski
|
||||
|
||||
**Wybrany temat:** Inteligentna śmieciarka
|
||||
|
||||
**Link do repozytorium projektu:** https://git.wmi.amu.edu.pl/s440556/SZI2019SmieciarzWmi
|
||||
|
||||
## Wybrany algorytm uczenia - drzewa decyzyjne
|
||||
|
||||
### Przygotowane dane
|
||||
|
||||
Aby zapewnić smieciarce jak najlepszy wynik, do przygotowania danych do uczenia wybrałem algorytm szukania najkrótszej ścieżki, który dawał najlepsze wyniki podczas projektu grupowego - **BestFS**.
|
||||
|
||||
Podczas każdego jednorazowego przebiegu algorytmu BestFS patrzyłem na to jaki krok śmieciarka wykonuje w danej sytuacji, a następnie dane kroki zapisywałem do pliku w formacie json, tworząc próbki do późniejszej nauki.
|
||||
|
||||
Przykładowa próbka w formacie json:
|
||||
|
||||
```json
|
||||
{
|
||||
"moveset": [
|
||||
{
|
||||
"maps": [[1, 1, 3, 4, 2, 2, 2, 2, 1], [2, 1, 1, 3, 1, 4, 1, 1, 1]],
|
||||
"moves": [1, 2]
|
||||
}
|
||||
]
|
||||
}
|
||||
```
|
||||
|
||||
`moveset` to tablica wszystkich próbek wykorzystywanych do nauki.
|
||||
Każdy element tablicy to obiekt posiadający dwa pola:
|
||||
`maps` - otoczenie śmieciarki w danym kroku,
|
||||
`moves` - ruch śmieciarki przy danym otoczeniu
|
||||
|
||||
W powyższym przykładzie dla czytelności, zostały przedstawione otoczenia 3x3 wokół śmieciarki. W implementacji obszar ten został powiększony do 7x7 w celu poprawienia dokładności algorytmu.
|
||||
|
||||
#### Maps
|
||||
|
||||
Spłaszczona tablicę dwuwymiarową przedstawiająca otoczenie śmieciarki w konkretnym momencie działania algorytmu. Każda z cyfr przedstawia inny obiekt na mapie:
|
||||
|
||||
- 1 - Trawa (Grass)
|
||||
- 2 - Droga (Road)
|
||||
- 3 - Wysypisko (Dump)
|
||||
- 4 - Dom (House)
|
||||
|
||||
Dla powyższego przykładu pierwsza sytuacja (`moveset[0].maps[0]`) przedstawia następujące otoczenie na mapie
|
||||
|
||||
```
|
||||
G G D
|
||||
H R R
|
||||
R R G
|
||||
```
|
||||
|
||||
#### Moves
|
||||
|
||||
Tablica ruchów śmieciarki. i-ty ruch w tablicy odpowiada i-temu otoczeniu. Wyróżnimay 5 różnych ruchów agenta:
|
||||
|
||||
- 1 - Lewo
|
||||
- 2 - Prawo
|
||||
- 3 - Dół
|
||||
- 4 - Góra
|
||||
- 99 - Zbierz śmieci
|
||||
|
||||
Tak więc dla powyższego otoczenia `1` będzie oznaczać, że agent ruszył się w lewo.
|
||||
|
||||
---
|
||||
|
||||
### Implementacja
|
||||
|
||||
Do implementacji uczenia poprzez drzewo decyzyjny wykorzystałem bibliotekę [scikit learn](https://scikit-learn.org) do języka **python**. Podając odpowiednie dane, biblioteka przygotuje nam model zdolny do samodzielnego poruszania się na mapie.
|
||||
|
||||
```python
|
||||
#Trenowanie modelu
|
||||
from sklearn import tree
|
||||
X = [Kolejne otoczenia 7x7 w danym kroku]
|
||||
Y = [Kolejne kroki odpowiednie dla danego otoczenia]
|
||||
clf = tree.DecisionTreeClassifier()
|
||||
clf = clf.fit(X, Y)
|
||||
|
||||
#Samodzielny ruch wytrenowanego modelu
|
||||
clf.predict([Otoczenie agenta])
|
||||
```
|
||||
|
||||
`clf.predict` zwróci nam 1 z 5 ruchów, które ma wykonać agent.
|
||||
|
||||
---
|
||||
|
||||
### Obserwacje
|
||||
|
||||
W idealnym przypadku wytrenowany model powinien odzwierciedlać algorytm BestFS, jako iż to na podstawie jego był trenowany i to jego decyzje starał się naśladować. W rzeczywistości jednak po przygotowaniu ok. 1000 próbek agent radził sobie różnorako. Na jednych mapach poruszał się dość sprawnie, jednak na wielu nie wiedział co ma robić. Przyczyny mogą być różne, jednak w mojej opinii, przygotowanych danych było jednak trochę za mało i gdyby dać o wiele więcej danych do wytrenowania modelu, rezultat byłby o wiele lepszy.
|
Before Width: | Height: | Size: 5.4 KiB |
Before Width: | Height: | Size: 1.7 KiB |
Before Width: | Height: | Size: 1.9 KiB |
Before Width: | Height: | Size: 1.5 KiB |
@ -1,11 +0,0 @@
|
||||
9 9
|
||||
2 1
|
||||
E E E H E E E E E
|
||||
E Y R R E H E E E
|
||||
E R E R E R E G E
|
||||
H R E R R R R R E
|
||||
H R E E R H E R E
|
||||
R R R R R E R R E
|
||||
E B R H E R R H E
|
||||
E E R R R R E E E
|
||||
E E H E H R R R H
|
@ -1,7 +0,0 @@
|
||||
6 5
|
||||
1 0
|
||||
Y R R R E H
|
||||
E R B R R R
|
||||
H R E R E R
|
||||
E R R R R G
|
||||
E H E H R H
|
@ -1,6 +0,0 @@
|
||||
13 4
|
||||
3 0
|
||||
E Y R R R H H R R H E E E
|
||||
H R R E R R R R R R R H E
|
||||
E E R E H E R H R E R R G
|
||||
H R R H E B R R R H E R H
|
@ -1,12 +0,0 @@
|
||||
12 10
|
||||
1 0
|
||||
Y R R R E H R R R R R E
|
||||
E R E R R E R E H E R H
|
||||
H R R H R R R R R R R E
|
||||
E E R E E E E R E E R H
|
||||
E E R R H E H R E E R E
|
||||
E E H R E E R R E H R E
|
||||
E R R R R R R H E R R R
|
||||
H R E E R H E E E R E G
|
||||
E R E H R E R R R R R R
|
||||
E R B R R R R E H E E H
|
@ -1,9 +0,0 @@
|
||||
7 7
|
||||
3 0
|
||||
E E E R E E E
|
||||
E E E R E E E
|
||||
E R R R R R E
|
||||
E R E E E R E
|
||||
E R E E E R E
|
||||
E R E E E R E
|
||||
H R R R R R H
|
@ -1,52 +0,0 @@
|
||||
from utilities import movement,check_moves
|
||||
from DataModels.House import House
|
||||
from DataModels.Dump import Dump
|
||||
from DataModels.Container import Container
|
||||
from config import GRID_WIDTH, GRID_HEIGHT
|
||||
from collections import deque
|
||||
|
||||
def BFS(grid, available_movement, gc_moveset, mode):
|
||||
queue = deque()
|
||||
visited_nodes=[]
|
||||
for x in range(GRID_WIDTH):
|
||||
visited_nodes.append([])
|
||||
for y in range(GRID_HEIGHT):
|
||||
visited_nodes[-1].append(0)
|
||||
visited_nodes[gc_moveset[-1][0]][gc_moveset[-1][1]] = 1
|
||||
queue.append([available_movement, gc_moveset, visited_nodes])
|
||||
|
||||
|
||||
while queue:
|
||||
possible_goals = []
|
||||
state = queue.popleft()
|
||||
avalible_movement_state = state[0]
|
||||
gc_moveset_state = state[1]
|
||||
visited_nodes_state = state[2]
|
||||
a = gc_moveset_state[-1][0]
|
||||
b = gc_moveset_state[-1][1]
|
||||
possible_goals.append([a+1,b])
|
||||
possible_goals.append([a-1,b])
|
||||
possible_goals.append([a,b+1])
|
||||
possible_goals.append([a,b-1])
|
||||
object_in_area = False
|
||||
for location in possible_goals:
|
||||
if GRID_WIDTH>location[0]>=0 and GRID_HEIGHT>location[1]>=0:
|
||||
cell = grid[location[0]][location[1]]
|
||||
if(type(cell) == mode and cell.unvisited):
|
||||
cell.unvisited = False
|
||||
object_in_area = True
|
||||
break
|
||||
|
||||
x,y = gc_moveset_state[-1]
|
||||
if(object_in_area):
|
||||
gc_moveset_state.append("pick_garbage")
|
||||
return (cell,[x,y], gc_moveset_state)
|
||||
|
||||
for direction in avalible_movement_state:
|
||||
x_next, y_next = movement(grid,x,y)[0][direction]
|
||||
if visited_nodes_state[x_next][y_next]==0:
|
||||
available_movement_next = check_moves(grid, x_next,y_next,direction)
|
||||
gc_moveset_next = gc_moveset_state.copy()
|
||||
gc_moveset_next.append([x_next,y_next])
|
||||
visited_nodes_state[x_next][y_next]=1
|
||||
queue.append([available_movement_next, gc_moveset_next,visited_nodes_state])
|
@ -1,68 +0,0 @@
|
||||
from utilities import movement,check_moves
|
||||
from DataModels.House import House
|
||||
from DataModels.Container import Container
|
||||
from config import GRID_WIDTH, GRID_HEIGHT
|
||||
from math import sqrt
|
||||
INF = float('Inf')
|
||||
|
||||
|
||||
def CalculateDistance(gc, object_list):
|
||||
min_distance_goal = ['-',INF]
|
||||
for h in object_list:
|
||||
distance = sqrt(pow(h[1][0]-gc[0],2)+pow(h[1][1]-gc[1],2))
|
||||
if(min_distance_goal[1] > distance):
|
||||
min_distance_goal = [h[1], distance]
|
||||
return min_distance_goal
|
||||
|
||||
def BestFS(grid, available_movement, gc_moveset, object_list, depth = 0):
|
||||
|
||||
x, y = gc_moveset[-1][0], gc_moveset[-1][1]
|
||||
|
||||
#calculate distance to the nearest object
|
||||
min_distance_goal = CalculateDistance([x,y], object_list)
|
||||
|
||||
if(min_distance_goal[1] == 1):
|
||||
gc_moveset.append("pick_garbage")
|
||||
cell = grid[min_distance_goal[0][0]][min_distance_goal[0][1]]
|
||||
object_list.remove([cell,min_distance_goal[0]])
|
||||
return([x, y], gc_moveset, object_list)
|
||||
|
||||
#if depth exceeded, return
|
||||
if(depth > 15 or len(available_movement) == 0):
|
||||
return
|
||||
|
||||
#set preffered directions based on the closest object
|
||||
preffered_directions = []
|
||||
discouraged_directions = []
|
||||
|
||||
if(min_distance_goal[0][0] > x):
|
||||
preffered_directions.append("right")
|
||||
if(min_distance_goal[0][0] < x):
|
||||
preffered_directions.append("left")
|
||||
if(min_distance_goal[0][1] > y):
|
||||
preffered_directions.append("down")
|
||||
if(min_distance_goal[0][1] < y):
|
||||
preffered_directions.append("up")
|
||||
|
||||
if(len(preffered_directions) == 1):
|
||||
discouraged_directions.append(movement(grid, x, y)[1][preffered_directions[0]])
|
||||
|
||||
#sort available moves according to preferences
|
||||
sorted = [o for o in preffered_directions if o in available_movement]
|
||||
for o in sorted:
|
||||
available_movement.remove(o)
|
||||
sorted.extend([o for o in available_movement if o not in discouraged_directions])
|
||||
for o in sorted:
|
||||
if(o in available_movement):
|
||||
available_movement.remove(o)
|
||||
sorted.extend(available_movement)
|
||||
available_movement = sorted.copy()
|
||||
|
||||
for direction in available_movement:
|
||||
x_next, y_next = movement(grid,x,y)[0][direction]
|
||||
available_movement_next = check_moves(grid, x_next,y_next,direction)
|
||||
gc_moveset_next = gc_moveset.copy()
|
||||
gc_moveset_next.append([x_next,y_next])
|
||||
result = BestFS(grid, available_movement_next, gc_moveset_next, object_list, depth + 1)
|
||||
if result!= None:
|
||||
return result
|
@ -1,39 +0,0 @@
|
||||
from utilities import movement,check_moves
|
||||
from DataModels.House import House
|
||||
from DataModels.Dump import Dump
|
||||
from DataModels.Container import Container
|
||||
from config import GRID_WIDTH, GRID_HEIGHT
|
||||
|
||||
def DFS(grid, available_movement, gc_moveset, mode,depth=0):
|
||||
|
||||
possible_goals = []
|
||||
a = gc_moveset[-1][0]
|
||||
b = gc_moveset[-1][1]
|
||||
possible_goals.append([a+1,b])
|
||||
possible_goals.append([a-1,b])
|
||||
possible_goals.append([a,b+1])
|
||||
possible_goals.append([a,b-1])
|
||||
object_in_area = False
|
||||
for location in possible_goals:
|
||||
if GRID_WIDTH>location[0]>=0 and GRID_HEIGHT>location[1]>=0:
|
||||
cell = grid[location[0]][location[1]]
|
||||
if(type(cell) == mode and cell.unvisited):
|
||||
cell.unvisited = False
|
||||
object_in_area = True
|
||||
break
|
||||
|
||||
x,y = gc_moveset[-1]
|
||||
if(object_in_area):
|
||||
gc_moveset.append("pick_garbage")
|
||||
return [cell,[x,y], gc_moveset]
|
||||
|
||||
if len(available_movement) == 0 or depth>30:
|
||||
return
|
||||
for direction in available_movement:
|
||||
x_next, y_next = movement(grid,x,y)[0][direction]
|
||||
available_movement_next = check_moves(grid, x_next,y_next,direction)
|
||||
gc_moveset_next = gc_moveset.copy()
|
||||
gc_moveset_next.append([x_next,y_next])
|
||||
result = DFS(grid, available_movement_next, gc_moveset_next,mode, depth+1)
|
||||
if result!= None:
|
||||
return result
|
39
config.py
@ -1,22 +1,27 @@
|
||||
import sys, random
|
||||
from MapGenerator import GenerateMap
|
||||
|
||||
CELL_SIZE = 64
|
||||
FPS = 60
|
||||
DELAY = 50
|
||||
|
||||
try:
|
||||
MAP_NAME = sys.argv[1]
|
||||
except:
|
||||
MAP_NAME = GenerateMap()
|
||||
import sys
|
||||
import getopt
|
||||
from sprites.cell import CELL_SIZE
|
||||
|
||||
|
||||
map = open( MAP_NAME, 'r' )
|
||||
def set_home_amount():
|
||||
arguments = sys.argv[1:]
|
||||
try:
|
||||
optlist, args = getopt.getopt(arguments, '', ['home-count='])
|
||||
for o, amount in optlist:
|
||||
if o == '--home-count':
|
||||
if int(amount) < 2:
|
||||
print('Home count too low - must be higher than 2')
|
||||
sys.exit(2)
|
||||
return int(amount)
|
||||
print('Missing argument: --home-count <amount>')
|
||||
sys.exit(2)
|
||||
except getopt.GetoptError as err:
|
||||
print(err)
|
||||
sys.exit(2)
|
||||
|
||||
GRID_WIDTH, GRID_HEIGHT = [int(x) for x in map.readline().split()]
|
||||
GC_X, GC_Y = [int(x) for x in map.readline().split()]
|
||||
|
||||
WINDOW_HEIGHT = GRID_HEIGHT * CELL_SIZE
|
||||
WINDOW_WIDTH = GRID_WIDTH * CELL_SIZE
|
||||
home_amount = set_home_amount()
|
||||
|
||||
HOUSE_CAPACITY = random.randint(1, 11)
|
||||
PLAY_WIDTH = (home_amount + 4)*CELL_SIZE
|
||||
PLAY_HEIGHT = PLAY_WIDTH
|
||||
HUD_HEIGHT = int(home_amount*CELL_SIZE/4)
|
||||
|
10
enums/house_image.py
Normal file
@ -0,0 +1,10 @@
|
||||
from enum import Enum
|
||||
class House_image(Enum):
|
||||
house = "images/house.png"
|
||||
plastic = "images/house_plastic.png"
|
||||
metal = "images/house_metal.png"
|
||||
glass = "images/house_glass.png"
|
||||
plastic_glass = "images/house_plastic_glass.png"
|
||||
plastic_metal = "images/house_plastic_metal.png"
|
||||
glass_metal = "images/house_glass_metal.png"
|
||||
full = "images/house_full.png"
|
BIN
fonts/Bazgroly.ttf
Normal file
68
game.py
Normal file
@ -0,0 +1,68 @@
|
||||
from pygame import *
|
||||
import sys
|
||||
import random
|
||||
from config import PLAY_WIDTH, PLAY_HEIGHT, HUD_HEIGHT, home_amount
|
||||
from sprites.house import House
|
||||
from sprites.hud import Hud
|
||||
from pygame.locals import *
|
||||
import utils
|
||||
|
||||
##INITIALIZE STATIC VARIABLES#########
|
||||
FPS = 20
|
||||
|
||||
all_sprites = sprite.Group()
|
||||
fps_clock = time.Clock()
|
||||
|
||||
######################################
|
||||
|
||||
interactables = {
|
||||
"homes": [],
|
||||
"landfills": []
|
||||
}
|
||||
|
||||
|
||||
##GAMEWINDOW##########################
|
||||
WINDOW_WIDTH = PLAY_WIDTH
|
||||
WINDOW_HEIGHT = PLAY_HEIGHT + HUD_HEIGHT
|
||||
GAMEWINDOW = display.set_mode((WINDOW_WIDTH, WINDOW_HEIGHT), 0, 32)
|
||||
hud = Hud(home_amount,WINDOW_WIDTH, WINDOW_HEIGHT,GAMEWINDOW)
|
||||
display.set_caption('Smieciarz WMI')
|
||||
icon = image.load('images/icon.png')
|
||||
display.set_icon(icon)
|
||||
######################################
|
||||
|
||||
##
|
||||
# Generate level
|
||||
utils.generate_grass(all_sprites)
|
||||
utils.generate_landfills(all_sprites, interactables)
|
||||
utils.generate_houses(all_sprites, interactables)
|
||||
gc = utils.generate_garbage_collector(all_sprites, interactables)
|
||||
##
|
||||
|
||||
##GAME LOOP#######################################################################
|
||||
while(1):
|
||||
for e in event.get():
|
||||
if e.type == QUIT:
|
||||
quit()
|
||||
sys.exit()
|
||||
if e.type == KEYUP:
|
||||
if e.key == K_UP:
|
||||
gc.move('up', interactables["homes"] + interactables["landfills"])
|
||||
if e.key == K_DOWN:
|
||||
gc.move('down', interactables["homes"] + interactables["landfills"])
|
||||
if e.key == K_RIGHT:
|
||||
gc.move('right', interactables["homes"] + interactables["landfills"])
|
||||
if e.key == K_LEFT:
|
||||
gc.move('left', interactables["homes"] + interactables["landfills"])
|
||||
|
||||
all_sprites.update()
|
||||
all_sprites.draw(GAMEWINDOW)
|
||||
|
||||
for item in all_sprites:
|
||||
if(type(item) == House):
|
||||
item.generate_rubbish()
|
||||
|
||||
hud.get_statistics(all_sprites)
|
||||
display.flip()
|
||||
fps_clock.tick(FPS)
|
||||
##################################################################################
|
BIN
images/garbage_collector.png
Normal file
After Width: | Height: | Size: 2.1 KiB |
BIN
images/grass.png
Normal file
After Width: | Height: | Size: 1.6 KiB |
BIN
images/house.png
Normal file
After Width: | Height: | Size: 2.3 KiB |
BIN
images/house_full.png
Normal file
After Width: | Height: | Size: 2.8 KiB |
BIN
images/house_glass.png
Normal file
After Width: | Height: | Size: 2.5 KiB |
BIN
images/house_glass_metal.png
Normal file
After Width: | Height: | Size: 2.7 KiB |
BIN
images/house_metal.png
Normal file
After Width: | Height: | Size: 2.5 KiB |
BIN
images/house_plastic.png
Normal file
After Width: | Height: | Size: 2.5 KiB |
BIN
images/house_plastic_glass.png
Normal file
After Width: | Height: | Size: 2.7 KiB |
BIN
images/house_plastic_metal.png
Normal file
After Width: | Height: | Size: 2.7 KiB |
BIN
images/icon.png
Normal file
After Width: | Height: | Size: 4.0 KiB |
Before Width: | Height: | Size: 5.3 KiB After Width: | Height: | Size: 5.3 KiB |
Before Width: | Height: | Size: 5.3 KiB After Width: | Height: | Size: 5.3 KiB |
Before Width: | Height: | Size: 5.2 KiB After Width: | Height: | Size: 5.2 KiB |
@ -1,63 +0,0 @@
|
||||
House 1 plastic,House 1 glass,House 1 metal,House 2 plastic,House 2 glass,House 2 metal,House 3 plastic,House 3 glass,House 3 metal,House 4 plastic,House 4 glass,House 4 metal,House 5 plastic,House 5 glass,House 5 metal,House 6 plastic,House 6 glass,House 6 metal
|
||||
8,4,10,0,0,1,8,9,10,1,7,7,8,5,7,8,2,1
|
||||
10,4,10,0,2,1,8,10,10,1,8,7,8,6,7,8,2,2
|
||||
10,4,10,0,3,2,10,10,10,1,9,8,10,6,7,9,3,4
|
||||
10,5,10,0,4,2,10,10,10,2,9,8,10,7,7,9,3,4
|
||||
10,5,10,0,4,3,10,10,10,2,10,8,10,7,9,9,3,4
|
||||
10,5,10,0,4,3,10,10,10,2,10,9,10,7,9,9,3,4
|
||||
10,5,10,0,4,4,10,10,10,2,10,9,10,7,10,9,4,5
|
||||
10,5,10,0,4,4,10,10,10,2,10,9,10,7,10,10,5,5
|
||||
10,5,10,2,5,4,10,10,10,3,10,9,10,7,10,10,5,6
|
||||
10,5,10,3,5,4,10,10,10,4,10,9,10,7,10,10,5,7
|
||||
10,5,10,3,6,4,10,10,10,6,10,9,10,10,10,10,6,7
|
||||
10,6,10,3,6,4,10,10,10,7,10,9,10,10,10,10,8,8
|
||||
10,7,10,3,8,5,10,10,10,8,10,10,10,10,10,10,8,9
|
||||
10,7,10,5,9,6,10,10,10,8,10,10,10,10,10,10,8,10
|
||||
10,7,10,6,9,6,10,10,10,9,10,10,10,10,10,10,8,10
|
||||
10,8,10,7,9,8,10,10,10,10,10,10,10,10,10,10,8,10
|
||||
10,9,10,8,9,10,10,10,10,10,10,10,10,10,10,10,8,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,8,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,8,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,9,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10
|
||||
10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10,10
|
|
@ -1,28 +0,0 @@
|
||||
Plastic left,Glass left,Metal left,GC plastic,GC glass,GC metal,Total collected
|
||||
18,27,26,0/10,0/10,0/10,0
|
||||
22,28,29,0/10,0/10,0/10,0
|
||||
23,33,33,0/10,0/10,0/10,0
|
||||
25,36,36,0/10,0/10,0/10,0
|
||||
27,39,37,0/10,0/10,0/10,0
|
||||
31,41,41,0/10,0/10,0/10,0
|
||||
36,43,41,0/10,0/10,0/10,0
|
||||
40,47,45,0/10,0/10,0/10,0
|
||||
41,51,45,0/10,0/10,0/10,0
|
||||
41,52,46,0/10,0/10,0/10,0
|
||||
43,54,48,0/10,0/10,0/10,0
|
||||
45,54,49,0/10,0/10,0/10,0
|
||||
48,57,50,0/10,0/10,0/10,0
|
||||
49,58,54,0/10,0/10,0/10,0
|
||||
51,59,54,0/10,0/10,0/10,0
|
||||
52,59,54,0/10,0/10,0/10,0
|
||||
54,59,55,0/10,0/10,0/10,0
|
||||
56,60,57,0/10,0/10,0/10,0
|
||||
57,60,58,0/10,0/10,0/10,0
|
||||
57,60,58,0/10,0/10,0/10,0
|
||||
57,60,58,0/10,0/10,0/10,0
|
||||
57,60,58,0/10,0/10,0/10,0
|
||||
57,60,59,0/10,0/10,0/10,0
|
||||
59,60,59,0/10,0/10,0/10,0
|
||||
59,60,60,0/10,0/10,0/10,0
|
||||
60,60,60,0/10,0/10,0/10,0
|
||||
60,60,60,0/10,0/10,0/10,0
|
|
@ -1,13 +0,0 @@
|
||||
Plastic left,Glass left,Metal left,GC plastic,GC glass,GC metal,Total collected
|
||||
20,33,20,0/10,0/10,0/10,0
|
||||
26,34,22,0/10,0/10,0/10,0
|
||||
27,37,27,0/10,0/10,0/10,0
|
||||
28,37,30,0/10,0/10,0/10,0
|
||||
30,40,33,0/10,0/10,0/10,0
|
||||
32,41,36,0/10,0/10,0/10,0
|
||||
35,42,38,0/10,0/10,0/10,0
|
||||
37,44,40,0/10,0/10,0/10,0
|
||||
39,45,41,0/10,0/10,0/10,0
|
||||
40,47,43,0/10,0/10,0/10,0
|
||||
42,47,45,0/10,0/10,0/10,0
|
||||
48,48,47,0/10,0/10,0/10,0
|
|
@ -1,9 +0,0 @@
|
||||
Plastic left,Glass left,Metal left,GC plastic,GC glass,GC metal,Total collected
|
||||
30,37,26,0/10,0/10,0/10,0
|
||||
34,39,27,0/10,0/10,0/10,0
|
||||
36,41,27,0/10,0/10,0/10,0
|
||||
40,43,29,0/10,0/10,0/10,0
|
||||
40,44,33,0/10,0/10,0/10,0
|
||||
40,45,34,0/10,0/10,0/10,0
|
||||
42,45,36,0/10,0/10,0/10,0
|
||||
43,46,38,0/10,0/10,0/10,0
|
|
@ -1,4 +0,0 @@
|
||||
Plastic left,Glass left,Metal left,GC plastic,GC glass,GC metal,Total collected
|
||||
21,37,28,0/10,0/10,0/10,0
|
||||
23,39,30,0/10,0/10,0/10,0
|
||||
25,40,30,0/10,0/10,0/10,0
|
|
@ -1,5 +0,0 @@
|
||||
Plastic left,Glass left,Metal left,GC plastic,GC glass,GC metal,Total collected
|
||||
32,32,30,0/10,0/10,0/10,0
|
||||
33,34,33,0/10,0/10,0/10,0
|
||||
34,36,34,0/10,0/10,0/10,0
|
||||
37,39,35,0/10,0/10,0/10,0
|
|
@ -1,144 +0,0 @@
|
||||
Plastic left,Glass left,Metal left,GC plastic,GC glass,GC metal,Total collected
|
||||
30,22,27,0/10,0/10,0/10,0
|
||||
33,27,29,0/10,0/10,0/10,0
|
||||
35,29,30,0/10,0/10,0/10,0
|
||||
35,34,32,0/10,0/10,0/10,0
|
||||
38,38,33,0/10,0/10,0/10,0
|
||||
40,41,33,0/10,0/10,0/10,0
|
||||
41,41,34,0/10,0/10,0/10,0
|
||||
43,43,36,0/10,0/10,0/10,0
|
||||
44,44,39,0/10,0/10,0/10,0
|
||||
47,45,41,0/10,0/10,0/10,0
|
||||
47,48,42,0/10,0/10,0/10,0
|
||||
47,48,45,0/10,0/10,0/10,0
|
||||
48,49,46,0/10,0/10,0/10,0
|
||||
49,49,46,0/10,0/10,0/10,0
|
||||
50,49,47,0/10,0/10,0/10,0
|
||||
50,49,47,0/10,0/10,0/10,0
|
||||
50,49,47,0/10,0/10,0/10,0
|
||||
50,50,48,0/10,0/10,0/10,0
|
||||
50,50,49,0/10,0/10,0/10,0
|
||||
50,50,49,0/10,0/10,0/10,0
|
||||
50,50,49,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
|
@ -1,51 +0,0 @@
|
||||
Plastic left,Glass left,Metal left,GC plastic,GC glass,GC metal,Total collected
|
||||
34,35,27,0/10,0/10,0/10,0
|
||||
36,37,27,0/10,0/10,0/10,0
|
||||
36,39,31,0/10,0/10,0/10,0
|
||||
38,41,31,0/10,0/10,0/10,0
|
||||
41,42,33,0/10,0/10,0/10,0
|
||||
44,42,33,0/10,0/10,0/10,0
|
||||
46,45,34,0/10,0/10,0/10,0
|
||||
46,47,36,0/10,0/10,0/10,0
|
||||
49,47,36,0/10,0/10,0/10,0
|
||||
49,47,38,0/10,0/10,0/10,0
|
||||
49,48,40,0/10,0/10,0/10,0
|
||||
50,49,40,0/10,0/10,0/10,0
|
||||
50,49,41,0/10,0/10,0/10,0
|
||||
50,49,41,0/10,0/10,0/10,0
|
||||
50,49,41,0/10,0/10,0/10,0
|
||||
50,50,41,0/10,0/10,0/10,0
|
||||
50,50,41,0/10,0/10,0/10,0
|
||||
50,50,44,0/10,0/10,0/10,0
|
||||
50,50,46,0/10,0/10,0/10,0
|
||||
50,50,46,0/10,0/10,0/10,0
|
||||
50,50,46,0/10,0/10,0/10,0
|
||||
50,50,47,0/10,0/10,0/10,0
|
||||
50,50,49,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
|
@ -1,26 +0,0 @@
|
||||
Plastic left,Glass left,Metal left,GC plastic,GC glass,GC metal,Total collected
|
||||
15,31,36,0/10,0/10,0/10,0
|
||||
19,36,39,0/10,0/10,0/10,0
|
||||
20,36,40,0/10,0/10,0/10,0
|
||||
24,38,40,0/10,0/10,0/10,0
|
||||
29,38,42,0/10,0/10,0/10,0
|
||||
32,38,45,0/10,0/10,0/10,0
|
||||
33,38,45,0/10,0/10,0/10,0
|
||||
33,39,46,0/10,0/10,0/10,0
|
||||
36,40,46,0/10,0/10,0/10,0
|
||||
38,40,46,0/10,0/10,0/10,0
|
||||
38,42,47,0/10,0/10,0/10,0
|
||||
39,44,49,0/10,0/10,0/10,0
|
||||
42,44,50,0/10,0/10,0/10,0
|
||||
42,45,50,0/10,0/10,0/10,0
|
||||
43,45,50,0/10,0/10,0/10,0
|
||||
43,46,50,0/10,0/10,0/10,0
|
||||
44,46,50,0/10,0/10,0/10,0
|
||||
44,46,50,0/10,0/10,0/10,0
|
||||
45,47,50,0/10,0/10,0/10,0
|
||||
45,49,50,0/10,0/10,0/10,0
|
||||
46,49,50,0/10,0/10,0/10,0
|
||||
47,50,50,0/10,0/10,0/10,0
|
||||
47,50,50,0/10,0/10,0/10,0
|
||||
48,50,50,0/10,0/10,0/10,0
|
||||
48,50,50,0/10,0/10,0/10,0
|
|
@ -1,9 +0,0 @@
|
||||
Plastic left,Glass left,Metal left,GC plastic,GC glass,GC metal,Total collected
|
||||
35,27,43,0/10,0/10,0/10,0
|
||||
37,28,45,0/10,0/10,0/10,0
|
||||
37,32,45,0/10,0/10,0/10,0
|
||||
39,35,46,0/10,0/10,0/10,0
|
||||
39,37,46,0/10,0/10,0/10,0
|
||||
40,37,46,0/10,0/10,0/10,0
|
||||
42,39,46,0/10,0/10,0/10,0
|
||||
44,39,46,0/10,0/10,0/10,0
|
|
@ -1,35 +0,0 @@
|
||||
Plastic left,Glass left,Metal left,GC plastic,GC glass,GC metal,Total collected
|
||||
25,15,21,0/10,0/10,0/10,0
|
||||
27,19,23,0/10,0/10,0/10,0
|
||||
29,22,28,0/10,0/10,0/10,0
|
||||
29,25,28,0/10,0/10,0/10,0
|
||||
36,29,30,0/10,0/10,0/10,0
|
||||
37,35,31,0/10,0/10,0/10,0
|
||||
38,38,33,0/10,0/10,0/10,0
|
||||
38,38,34,0/10,0/10,0/10,0
|
||||
38,39,36,0/10,0/10,0/10,0
|
||||
41,43,36,0/10,0/10,0/10,0
|
||||
43,44,37,0/10,0/10,0/10,0
|
||||
45,46,39,0/10,0/10,0/10,0
|
||||
45,48,39,0/10,0/10,0/10,0
|
||||
46,49,42,0/10,0/10,0/10,0
|
||||
47,49,45,0/10,0/10,0/10,0
|
||||
48,49,45,0/10,0/10,0/10,0
|
||||
49,50,46,0/10,0/10,0/10,0
|
||||
50,50,46,0/10,0/10,0/10,0
|
||||
50,50,46,0/10,0/10,0/10,0
|
||||
50,50,46,0/10,0/10,0/10,0
|
||||
50,50,47,0/10,0/10,0/10,0
|
||||
50,50,49,0/10,0/10,0/10,0
|
||||
50,50,49,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
|
@ -1,17 +0,0 @@
|
||||
Plastic left,Glass left,Metal left,GC plastic,GC glass,GC metal,Total collected
|
||||
35,23,40,0/10,0/10,0/10,0
|
||||
36,28,40,0/10,0/10,0/10,0
|
||||
41,30,40,0/10,0/10,0/10,0
|
||||
43,31,42,0/10,0/10,0/10,0
|
||||
44,34,42,0/10,0/10,0/10,0
|
||||
45,36,46,0/10,0/10,0/10,0
|
||||
48,36,47,0/10,0/10,0/10,0
|
||||
48,38,47,0/10,0/10,0/10,0
|
||||
48,39,49,0/10,0/10,0/10,0
|
||||
48,42,49,0/10,0/10,0/10,0
|
||||
49,44,49,0/10,0/10,0/10,0
|
||||
50,46,49,0/10,0/10,0/10,0
|
||||
50,47,49,0/10,0/10,0/10,0
|
||||
50,48,50,0/10,0/10,0/10,0
|
||||
50,49,50,0/10,0/10,0/10,0
|
||||
50,49,50,0/10,0/10,0/10,0
|
|
@ -1,10 +0,0 @@
|
||||
Plastic left,Glass left,Metal left,GC plastic,GC glass,GC metal,Total collected
|
||||
27,34,21,0/10,0/10,0/10,0
|
||||
29,35,21,0/10,0/10,0/10,0
|
||||
31,36,22,0/10,0/10,0/10,0
|
||||
33,39,22,0/10,0/10,0/10,0
|
||||
34,44,23,0/10,0/10,0/10,0
|
||||
37,45,26,0/10,0/10,0/10,0
|
||||
38,45,27,0/10,0/10,0/10,0
|
||||
42,46,30,0/10,0/10,0/10,0
|
||||
42,47,33,0/10,0/10,0/10,0
|
|
@ -1,8 +0,0 @@
|
||||
Plastic left,Glass left,Metal left,GC plastic,GC glass,GC metal,Total collected
|
||||
13,25,23,0/10,0/10,0/10,0
|
||||
17,28,27,0/10,0/10,0/10,0
|
||||
20,33,30,0/10,0/10,0/10,0
|
||||
23,37,32,0/10,0/10,0/10,0
|
||||
25,38,35,0/10,0/10,0/10,0
|
||||
27,41,35,0/10,0/10,0/10,0
|
||||
28,42,37,0/10,0/10,0/10,0
|
|
@ -1,8 +0,0 @@
|
||||
Plastic left,Glass left,Metal left,GC plastic,GC glass,GC metal,Total collected
|
||||
25,8,17,0/10,0/10,0/10,0
|
||||
28,9,18,0/10,0/10,0/10,0
|
||||
32,10,18,0/10,0/10,0/10,0
|
||||
35,12,21,0/10,0/10,0/10,0
|
||||
37,13,23,0/10,0/10,0/10,0
|
||||
39,16,24,0/10,0/10,0/10,0
|
||||
40,20,26,0/10,0/10,0/10,0
|
|
@ -1,7 +0,0 @@
|
||||
Plastic left,Glass left,Metal left,GC plastic,GC glass,GC metal,Total collected
|
||||
21,18,22,0/10,0/10,0/10,0
|
||||
22,20,25,0/10,0/10,0/10,0
|
||||
25,22,31,0/10,0/10,0/10,0
|
||||
25,27,35,0/10,0/10,0/10,0
|
||||
28,29,36,0/10,0/10,0/10,0
|
||||
31,32,39,0/10,0/10,0/10,0
|
|
@ -1,6 +0,0 @@
|
||||
Plastic left,Glass left,Metal left,GC plastic,GC glass,GC metal,Total collected
|
||||
39,20,30,0/10,0/10,0/10,0
|
||||
41,21,30,0/10,0/10,0/10,0
|
||||
42,22,32,0/10,0/10,0/10,0
|
||||
43,23,35,0/10,0/10,0/10,0
|
||||
45,25,37,0/10,0/10,0/10,0
|
|
@ -1,3 +0,0 @@
|
||||
Plastic left,Glass left,Metal left,GC plastic,GC glass,GC metal,Total collected
|
||||
21,23,5,0/10,0/10,0/10,0
|
||||
21,24,5,0/10,0/10,0/10,0
|
|
@ -1,31 +0,0 @@
|
||||
Plastic left,Glass left,Metal left,GC plastic,GC glass,GC metal,Total collected
|
||||
33,26,21,0/10,0/10,0/10,0
|
||||
35,28,23,0/10,0/10,0/10,0
|
||||
39,28,27,0/10,0/10,0/10,0
|
||||
40,28,32,0/10,0/10,0/10,0
|
||||
41,31,34,0/10,0/10,0/10,0
|
||||
42,32,35,0/10,0/10,0/10,0
|
||||
45,32,35,0/10,0/10,0/10,0
|
||||
47,34,36,0/10,0/10,0/10,0
|
||||
47,34,37,0/10,0/10,0/10,0
|
||||
47,34,40,0/10,0/10,0/10,0
|
||||
48,36,41,0/10,0/10,0/10,0
|
||||
48,37,43,0/10,0/10,0/10,0
|
||||
49,39,46,0/10,0/10,0/10,0
|
||||
49,40,47,0/10,0/10,0/10,0
|
||||
49,40,48,0/10,0/10,0/10,0
|
||||
50,40,49,0/10,0/10,0/10,0
|
||||
50,42,50,0/10,0/10,0/10,0
|
||||
50,42,50,0/10,0/10,0/10,0
|
||||
50,44,50,0/10,0/10,0/10,0
|
||||
50,45,50,0/10,0/10,0/10,0
|
||||
50,45,50,0/10,0/10,0/10,0
|
||||
50,45,50,0/10,0/10,0/10,0
|
||||
50,45,50,0/10,0/10,0/10,0
|
||||
50,46,50,0/10,0/10,0/10,0
|
||||
50,46,50,0/10,0/10,0/10,0
|
||||
50,46,50,0/10,0/10,0/10,0
|
||||
50,46,50,0/10,0/10,0/10,0
|
||||
50,46,50,0/10,0/10,0/10,0
|
||||
50,46,50,0/10,0/10,0/10,0
|
||||
50,46,50,0/10,0/10,0/10,0
|
|
@ -1,14 +0,0 @@
|
||||
Plastic left,Glass left,Metal left,GC plastic,GC glass,GC metal,Total collected
|
||||
29,15,46,0/10,0/10,0/10,0
|
||||
31,20,46,0/10,0/10,0/10,0
|
||||
33,20,46,0/10,0/10,0/10,0
|
||||
35,23,47,0/10,0/10,0/10,0
|
||||
37,25,47,0/10,0/10,0/10,0
|
||||
38,27,47,0/10,0/10,0/10,0
|
||||
39,31,47,0/10,0/10,0/10,0
|
||||
40,31,47,0/10,0/10,0/10,0
|
||||
41,32,47,0/10,0/10,0/10,0
|
||||
42,34,47,0/10,0/10,0/10,0
|
||||
45,38,48,0/10,0/10,0/10,0
|
||||
48,41,48,0/10,0/10,0/10,0
|
||||
48,42,49,0/10,0/10,0/10,0
|
|
@ -1,692 +0,0 @@
|
||||
Plastic left,Glass left,Metal left,GC plastic,GC glass,GC metal,Total collected
|
||||
26,15,30,0/10,0/10,0/10,0
|
||||
28,19,31,0/10,0/10,0/10,0
|
||||
31,24,37,0/10,0/10,0/10,0
|
||||
33,27,41,0/10,0/10,0/10,0
|
||||
34,29,41,0/10,0/10,0/10,0
|
||||
35,31,42,0/10,0/10,0/10,0
|
||||
37,34,44,0/10,0/10,0/10,0
|
||||
38,36,44,0/10,0/10,0/10,0
|
||||
38,38,46,0/10,0/10,0/10,0
|
||||
38,38,46,0/10,0/10,0/10,0
|
||||
39,40,47,0/10,0/10,0/10,0
|
||||
41,41,48,0/10,0/10,0/10,0
|
||||
41,45,48,0/10,0/10,0/10,0
|
||||
42,46,49,0/10,0/10,0/10,0
|
||||
44,49,49,0/10,0/10,0/10,0
|
||||
45,49,49,0/10,0/10,0/10,0
|
||||
47,49,49,0/10,0/10,0/10,0
|
||||
48,49,49,0/10,0/10,0/10,0
|
||||
49,49,50,0/10,0/10,0/10,0
|
||||
50,49,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
||||
50,50,50,0/10,0/10,0/10,0
|
|
@ -1,63 +0,0 @@
|
||||
Plastic left,Glass left,Metal left,GC plastic,GC glass,GC metal,Total collected
|
||||
33,27,36,0/10,0/10,0/10,0
|
||||
35,32,37,0/10,0/10,0/10,0
|
||||
40,35,41,0/10,0/10,0/10,0
|
||||
41,38,41,0/10,0/10,0/10,0
|
||||
41,39,44,0/10,0/10,0/10,0
|
||||
41,39,45,0/10,0/10,0/10,0
|
||||
41,40,48,0/10,0/10,0/10,0
|
||||
42,41,48,0/10,0/10,0/10,0
|
||||
45,42,49,0/10,0/10,0/10,0
|
||||
47,42,50,0/10,0/10,0/10,0
|
||||
49,47,50,0/10,0/10,0/10,0
|
||||
50,50,51,0/10,0/10,0/10,0
|
||||
51,53,54,0/10,0/10,0/10,0
|
||||
53,54,56,0/10,0/10,0/10,0
|
||||
55,54,56,0/10,0/10,0/10,0
|
||||
57,55,58,0/10,0/10,0/10,0
|
||||
58,56,60,0/10,0/10,0/10,0
|
||||
60,58,60,0/10,0/10,0/10,0
|
||||
60,58,60,0/10,0/10,0/10,0
|
||||
60,59,60,0/10,0/10,0/10,0
|
||||
60,60,60,0/10,0/10,0/10,0
|
||||
60,60,60,0/10,0/10,0/10,0
|
||||
60,60,60,0/10,0/10,0/10,0
|
||||
60,60,60,0/10,0/10,0/10,0
|
||||
60,60,60,0/10,0/10,0/10,0
|
||||
60,60,60,0/10,0/10,0/10,0
|
||||
60,60,60,0/10,0/10,0/10,0
|
||||
60,60,60,0/10,0/10,0/10,0
|
||||
60,60,60,0/10,0/10,0/10,0
|
||||
60,60,60,0/10,0/10,0/10,0
|
||||
60,60,60,0/10,0/10,0/10,0
|
||||
60,60,60,0/10,0/10,0/10,0
|
||||
60,60,60,0/10,0/10,0/10,0
|
||||
60,60,60,0/10,0/10,0/10,0
|
||||
60,60,60,0/10,0/10,0/10,0
|
||||
60,60,60,0/10,0/10,0/10,0
|
||||
60,60,60,0/10,0/10,0/10,0
|
||||
60,60,60,0/10,0/10,0/10,0
|
||||
60,60,60,0/10,0/10,0/10,0
|
||||
60,60,60,0/10,0/10,0/10,0
|
||||
60,60,60,0/10,0/10,0/10,0
|
||||
60,60,60,0/10,0/10,0/10,0
|
||||
60,60,60,0/10,0/10,0/10,0
|
||||
60,60,60,0/10,0/10,0/10,0
|
||||
60,60,60,0/10,0/10,0/10,0
|
||||
60,60,60,0/10,0/10,0/10,0
|
||||
60,60,60,0/10,0/10,0/10,0
|
||||
60,60,60,0/10,0/10,0/10,0
|
||||
60,60,60,0/10,0/10,0/10,0
|
||||
60,60,60,0/10,0/10,0/10,0
|
||||
60,60,60,0/10,0/10,0/10,0
|
||||
60,60,60,0/10,0/10,0/10,0
|
||||
60,60,60,0/10,0/10,0/10,0
|
||||
60,60,60,0/10,0/10,0/10,0
|
||||
60,60,60,0/10,0/10,0/10,0
|
||||
60,60,60,0/10,0/10,0/10,0
|
||||
60,60,60,0/10,0/10,0/10,0
|
||||
60,60,60,0/10,0/10,0/10,0
|
||||
60,60,60,0/10,0/10,0/10,0
|
||||
60,60,60,0/10,0/10,0/10,0
|
||||
60,60,60,0/10,0/10,0/10,0
|
||||
60,60,60,0/10,0/10,0/10,0
|
|
122
main.py
@ -1,122 +0,0 @@
|
||||
#!linux_env/bin/python3
|
||||
|
||||
import pygame
|
||||
import sys
|
||||
from random import randint
|
||||
from config import WINDOW_HEIGHT, WINDOW_WIDTH, GRID_HEIGHT, GRID_WIDTH, HOUSE_CAPACITY, FPS, GC_X, GC_Y, MAP_NAME
|
||||
|
||||
from PIL import Image,ImageDraw
|
||||
|
||||
from DataModels.Grass import Grass
|
||||
from DataModels.House import House
|
||||
from DataModels.Dump import Dump
|
||||
from DataModels.Road import Road
|
||||
from DataModels.GC import GC
|
||||
|
||||
pygame.init()
|
||||
|
||||
pygame_sprites = pygame.sprite.Group()
|
||||
house_count=0
|
||||
dump_count=0
|
||||
FPS_CLOCK = pygame.time.Clock()
|
||||
GAME_WINDOW = pygame.display.set_mode((WINDOW_WIDTH, WINDOW_HEIGHT), 0, 32)
|
||||
|
||||
map = open(MAP_NAME, 'r')
|
||||
map.readline()
|
||||
map.readline()
|
||||
|
||||
map_objects = [[None for y in range(0, GRID_HEIGHT)]
|
||||
for x in range(0, GRID_WIDTH)]
|
||||
|
||||
|
||||
def generate(letter):
|
||||
key = 'D' if letter in ['B', 'G', 'Y'] else letter
|
||||
letter_mapping = {
|
||||
'E': lambda x, y: Grass(x, y),
|
||||
'H': lambda x, y, max_rubbish, yellow, green, blue: House(x, y, max_rubbish, yellow, green, blue),
|
||||
'D': lambda x, y, max_rubbish, dump_type: Dump(x, y, max_rubbish, dump_type),
|
||||
'R': lambda x, y: Road(x, y)
|
||||
}
|
||||
|
||||
return letter_mapping[key]
|
||||
|
||||
|
||||
i = 0
|
||||
for y in map.readlines():
|
||||
for x in y.split():
|
||||
|
||||
x_coord = i % GRID_WIDTH
|
||||
y_coord = (i-x_coord)//GRID_WIDTH
|
||||
|
||||
yellow, green, blue = [randint(0, HOUSE_CAPACITY // 2), randint(
|
||||
0, HOUSE_CAPACITY // 2), randint(0, HOUSE_CAPACITY // 2)]
|
||||
if x is 'E':
|
||||
map_objects[x_coord][y_coord] = generate(x)(x_coord, y_coord)
|
||||
elif x is 'H':
|
||||
map_objects[x_coord][y_coord] = generate(x)(
|
||||
x_coord, y_coord, HOUSE_CAPACITY, yellow, green, blue)
|
||||
house_count+=1
|
||||
elif x is 'B':
|
||||
map_objects[x_coord][y_coord] = generate(
|
||||
x)(x_coord, y_coord, 100, "Dump_Blue")
|
||||
dump_count+=1
|
||||
elif x is 'G':
|
||||
map_objects[x_coord][y_coord] = generate(
|
||||
x)(x_coord, y_coord, 100, "Dump_Green")
|
||||
dump_count+=1
|
||||
elif x is 'Y':
|
||||
map_objects[x_coord][y_coord] = generate(
|
||||
x)(x_coord, y_coord, 100, "Dump_Yellow")
|
||||
dump_count+=1
|
||||
elif x is 'R':
|
||||
map_objects[x_coord][y_coord] = generate(x)(x_coord, y_coord)
|
||||
i += 1
|
||||
|
||||
|
||||
for line in map_objects:
|
||||
for item in line:
|
||||
pygame_sprites.add(item)
|
||||
|
||||
gc = GC(GC_X, GC_Y, 200)
|
||||
print("GC: " + str(GC_X) + str(GC_Y))
|
||||
pygame_sprites.add(gc)
|
||||
|
||||
while True:
|
||||
|
||||
for event in pygame.event.get():
|
||||
if event.type == pygame.QUIT:
|
||||
pygame.quit()
|
||||
sys.exit()
|
||||
elif event.type == pygame.KEYUP:
|
||||
if event.key == pygame.K_UP:
|
||||
gc.move("up", map_objects)
|
||||
elif event.key == pygame.K_DOWN:
|
||||
gc.move("down", map_objects)
|
||||
elif event.key == pygame.K_LEFT:
|
||||
gc.move("left", map_objects)
|
||||
elif event.key == pygame.K_RIGHT:
|
||||
gc.move("right", map_objects)
|
||||
elif event.key == pygame.K_SPACE:
|
||||
gc.collect(map_objects)
|
||||
elif event.key == pygame.K_0:
|
||||
gc.find_houses(map_objects,house_count,dump_count, "DFS")
|
||||
elif event.key == pygame.K_9:
|
||||
gc.find_houses_BestFS(map_objects)
|
||||
elif event.key == pygame.K_8:
|
||||
gc.find_houses(map_objects,house_count,dump_count, "BFS")
|
||||
|
||||
gc.make_actions_from_list(map_objects)
|
||||
pygame_sprites.update()
|
||||
pygame_sprites.draw(GAME_WINDOW)
|
||||
|
||||
#draw GC moves
|
||||
bg_rect = pygame.Surface((105,30), pygame.SRCALPHA)
|
||||
bg_rect.fill((0,0,0,160))
|
||||
GAME_WINDOW.blit(bg_rect, (0, WINDOW_HEIGHT-30))
|
||||
|
||||
font = pygame.font.SysFont("monospace", 15)
|
||||
gc_moves = font.render("Moves: " + str(gc.get_moves_count()), 1, (255,255,255))
|
||||
GAME_WINDOW.blit(gc_moves, (10, WINDOW_HEIGHT - 25))
|
||||
|
||||
pygame.display.flip()
|
||||
FPS_CLOCK.tick(FPS)
|
@ -1,22 +0,0 @@
|
||||
:- [house_positions].
|
||||
|
||||
check_position(X, Y):-
|
||||
collectable_object(Z,X,Y),
|
||||
write(Z),nl.
|
||||
|
||||
full(Collectable_object, Material) :-
|
||||
contains(Collectable_object, Material,10).
|
||||
|
||||
contains(Collectable_object, Material):-
|
||||
contains(Collectable_object,Material,Y),
|
||||
Y>0.
|
||||
|
||||
contains(Collectable_object) :-
|
||||
contains(Collectable_object, paper);
|
||||
contains(Collectable_object, glass);
|
||||
contains(Collectable_object, metal).
|
||||
|
||||
full(Collectable_object) :-
|
||||
full(Collectable_object, paper),
|
||||
full(Collectable_object, metal),
|
||||
full(Collectable_object, glass).
|
@ -1,9 +0,0 @@
|
||||
collectable_object(landfill, 0, 0).
|
||||
collectable_object(landfill, 1, 0).
|
||||
collectable_object(landfill, 2, 0).
|
||||
collectable_object(house, 8, 6).
|
||||
collectable_object(house, 6, 8).
|
||||
collectable_object(house, 2, 6).
|
||||
collectable_object(house, 8, 8).
|
||||
collectable_object(house, 9, 2).
|
||||
collectable_object(house, 5, 3).
|
@ -66,29 +66,29 @@ Na ten moment na planszy pojawiają się instancje klas:
|
||||
|
||||
**Struktura plików projektu**
|
||||
|
||||
--enums => Klasy dziedziczące po klasie *Enum*, ułatwiające parsowanie informacji
|
||||
--enums => Klasy dziedziczące po klasie *Enum*, ułatwiające parsowanie informacji
|
||||
|
||||
--fonts => Czcionki
|
||||
--fonts => Czcionki
|
||||
|
||||
--images => Obrazy i ikony używane w aplikacji
|
||||
--images => Obrazy i ikony używane w aplikacji
|
||||
|
||||
--raports => Raporty
|
||||
--raports => Raporty
|
||||
|
||||
--sprites => Klasy reprezentujące obiekty na mapie
|
||||
--sprites => Klasy reprezentujące obiekty na mapie
|
||||
|
||||
.gitignore
|
||||
.gitignore
|
||||
|
||||
config.py => Plik przechowujący funkcję zarządzające konfiguracją aplikacji
|
||||
config.py => Plik przechowujący funkcję zarządzające konfiguracją aplikacji
|
||||
|
||||
game.py => Plik rozruchowy programu
|
||||
game.py => Plik rozruchowy programu
|
||||
|
||||
utils.py => Funkcje pomocnicze
|
||||
utils.py => Funkcje pomocnicze
|
||||
|
||||
README.md => Informacje o aplikacji
|
||||
README.md => Informacje o aplikacji
|
||||
|
||||
requirements.txt => Przechowuje informacje na temat używanych bibliotek
|
||||
requirements.txt => Przechowuje informacje na temat używanych bibliotek
|
||||
|
||||
to_do.txt => Lista przyszłych zadań do zrobienia
|
||||
to_do.txt => Lista przyszłych zadań do zrobienia
|
||||
|
||||
|
||||
|
||||
@ -136,3 +136,4 @@ env\Scripts\pip.exe install -r requirements.txt
|
||||
env\Scripts\python.exe ./game.py --home-count=amount
|
||||
```
|
||||
|
||||
|
@ -1,2 +1 @@
|
||||
Pillow==5.4.1
|
||||
pygame==1.9.5
|
||||
pygame==1.9.4
|
||||
|
18
sprites/cell.py
Normal file
@ -0,0 +1,18 @@
|
||||
# -*- coding: utf-8 -*-
|
||||
import pygame
|
||||
import sys
|
||||
from pygame.locals import *
|
||||
|
||||
CELL_SIZE = 64
|
||||
|
||||
|
||||
class Cell(pygame.sprite.Sprite):
|
||||
def __init__(self, x, y):
|
||||
pygame.sprite.Sprite.__init__(self)
|
||||
self.x = x
|
||||
self.y = y
|
||||
self.update()
|
||||
|
||||
def update(self):
|
||||
self.rect = pygame.Rect(
|
||||
self.x*CELL_SIZE, self.y*CELL_SIZE, CELL_SIZE, CELL_SIZE)
|
58
sprites/garbage_collector.py
Normal file
@ -0,0 +1,58 @@
|
||||
import pygame
|
||||
from sprites.cell import Cell, CELL_SIZE
|
||||
from sprites.house import House
|
||||
from config import PLAY_HEIGHT, PLAY_WIDTH
|
||||
|
||||
class Garbage_collector(Cell):
|
||||
def __init__(self, x, y):
|
||||
GC_CAPACITY = 10
|
||||
|
||||
Cell.__init__(self, x, y)
|
||||
self.image = pygame.image.load("images/garbage_collector.png")
|
||||
self.move_options = {
|
||||
"up": lambda forbidden: ('y', self.y - 1) if (self.x, self.y - 1) not in forbidden and self.y - 1 >= 0 else ('y', self.y),
|
||||
"down": lambda forbidden: ('y', self.y + 1) if (self.x, self.y + 1) not in forbidden and self.y + 1 < PLAY_HEIGHT // CELL_SIZE else ('y', self.y),
|
||||
"left": lambda forbidden: ('x', self.x - 1) if (self.x - 1, self.y) not in forbidden and self.x - 1 >= 0 else ('x', self.x),
|
||||
"right": lambda forbidden: ('x', self.x + 1) if (self.x + 1, self.y) not in forbidden and self.x + 1 < PLAY_WIDTH // CELL_SIZE else ('x', self.x)
|
||||
}
|
||||
self.trash_space_taken = {
|
||||
"plastic": 0,
|
||||
"glass": 0,
|
||||
"metal": 0
|
||||
}
|
||||
self.trash_collected = 0
|
||||
|
||||
def move(self, direction, forbidden):
|
||||
(destination, value) = self.move_options[direction](forbidden)
|
||||
if(destination is 'x'):
|
||||
self.x = value
|
||||
elif(destination is 'y'):
|
||||
self.y = value
|
||||
self.update()
|
||||
|
||||
def collect_trash(self, house):
|
||||
rubbish = house.get_rubbish_data()
|
||||
to_collect = rubbish
|
||||
|
||||
if(rubbish[0] > GC_CAPACITY - self.trash_space_taken.get("plastic")):
|
||||
to_collect[0] = self.trash_space_taken.get("plastic")
|
||||
self.trash_space_taken['plastic'] += to_collect[0]
|
||||
self.trash_collected += to_collect[0]
|
||||
|
||||
if(rubbish[1] > GC_CAPACITY - self.trash_space_taken.get("glass")):
|
||||
to_collect[1] = self.trash_space_taken.get("glass")
|
||||
self.trash_space_taken['glass'] += to_collect[1]
|
||||
self.trash_collected += to_collect[1]
|
||||
|
||||
if(rubbish[2] > GC_CAPACITY - self.trash_space_taken.get("metal")):
|
||||
to_collect[2] = self.trash_space_taken.get("metal")
|
||||
self.trash_space_taken['metal'] += to_collect[2]
|
||||
self.trash_collected += to_collect[2]
|
||||
|
||||
house.give_away_rubbish(to_collect[0], to_collect[1], to_collect[2])
|
||||
|
||||
def get_collect_data(self):
|
||||
return self.trash_collected
|
||||
|
||||
def get_space_data(self):
|
||||
return self.trash_space_taken
|
9
sprites/grass.py
Normal file
@ -0,0 +1,9 @@
|
||||
import pygame
|
||||
import sys
|
||||
from sprites.cell import Cell
|
||||
|
||||
|
||||
class Grass(Cell):
|
||||
def __init__(self, x, y):
|
||||
Cell.__init__(self, x, y)
|
||||
self.image = pygame.image.load("images/grass.png")
|
58
sprites/house.py
Normal file
@ -0,0 +1,58 @@
|
||||
import pygame
|
||||
import sys
|
||||
import random
|
||||
from enum import Enum
|
||||
from sprites.cell import Cell
|
||||
from enums.house_image import House_image
|
||||
|
||||
PLASTIC = 0 # blue
|
||||
GLASS = 1 # green
|
||||
METAL = 2 # yellow
|
||||
|
||||
|
||||
class House(Cell):
|
||||
def __init__(self, x, y, max_plastic, max_glass, max_metal):
|
||||
Cell.__init__(self, x, y)
|
||||
self.image = pygame.image.load(House_image.house.value)
|
||||
self.rubbish = [random.randint(0, max_plastic), random.randint(
|
||||
0, max_glass), random.randint(0, max_metal)] # plastic, glass, metal
|
||||
|
||||
self.max_plastic = max_plastic
|
||||
self.max_glass = max_glass
|
||||
self.max_metal = max_metal
|
||||
|
||||
def generate_rubbish(self):
|
||||
if(random.randint(0, 25) == 1): # 1/25 szansa na wyrzucenie śmiecia w klatce
|
||||
thrash_type = random.randint(0, 2)
|
||||
self.rubbish[thrash_type] = self.rubbish[thrash_type] + 1
|
||||
|
||||
#mozna ladniej?
|
||||
if(self.rubbish[PLASTIC] > self.max_plastic):
|
||||
if(self.rubbish[GLASS] > self.max_glass):
|
||||
if(self.rubbish[METAL] > self.max_metal):
|
||||
self.image = pygame.image.load(House_image.full.value) #plastik, szklo, metal
|
||||
else:
|
||||
self.image = pygame.image.load(House_image.plastic_glass.value) #plastik, szklo
|
||||
elif(self.rubbish[METAL] > self.max_metal):
|
||||
self.image = pygame.image.load(House_image.plastic_metal.value) #plastik, metal
|
||||
else:
|
||||
self.image = pygame.image.load(House_image.plastic.value) #plastik
|
||||
elif(self.rubbish[GLASS] > self.max_glass):
|
||||
if(self.rubbish[METAL] > self.max_metal):
|
||||
self.image = pygame.image.load(House_image.glass_metal.value) #szklo, metal
|
||||
else:
|
||||
self.image = pygame.image.load(House_image.glass.value) #szklo
|
||||
elif(self.rubbish[METAL] > self.max_metal):
|
||||
self.image = pygame.image.load(House_image.metal.value) #metal
|
||||
|
||||
def give_away_rubbish(self, plastic, glass, metal):
|
||||
self.rubbish[PLASTIC] -= plastic
|
||||
self.rubbish[GLASS] -= glass
|
||||
self.rubbish[METAL] -= metal
|
||||
|
||||
def check_rubbish_status(self):
|
||||
print("plastic: " + str(self.rubbish[PLASTIC]) + " glass: " + str(
|
||||
self.rubbish[GLASS]) + " metal: " + str(self.rubbish[METAL]))
|
||||
|
||||
def get_rubbish_data(self):
|
||||
return self.rubbish
|
56
sprites/hud.py
Normal file
@ -0,0 +1,56 @@
|
||||
import pygame
|
||||
from sprites.house import House
|
||||
from sprites.garbage_collector import Garbage_collector
|
||||
from config import HUD_HEIGHT
|
||||
HUD_COLOR = (51,21,4)
|
||||
WHITE = (255,255,255)
|
||||
class Hud():
|
||||
texts = []
|
||||
def __init__(self,house_amount,WINDOW_WIDTH, WINDOW_HEIGHT,GAMEWINDOW):
|
||||
pygame.init()
|
||||
hud_upper = WINDOW_WIDTH - HUD_HEIGHT
|
||||
hud_lower = WINDOW_WIDTH
|
||||
height = hud_upper - hud_lower
|
||||
font_type = 'fonts/Bazgroly.ttf'
|
||||
font_size = house_amount * 2
|
||||
GAMEWINDOW.fill(HUD_COLOR)
|
||||
font = pygame.font.Font(font_type, font_size)
|
||||
|
||||
gc_plastic_text = font.render("Plastic: 0/0",True,WHITE)
|
||||
gc_metal_text = font.render("Metal: 0/0",True,WHITE)
|
||||
gc_glass_text = font.render("Glass: 0/0",True,WHITE)
|
||||
map_plastic_text = font.render("Plastic: 0",True,WHITE)
|
||||
map_metal_text = font.render("Metal: 0",True,WHITE)
|
||||
map_glass_text = font.render("Glass: 0",True,WHITE)
|
||||
overall_text = font.render("Garbage thrown away: 0",True,WHITE)
|
||||
GAMEWINDOW.blit(overall_text,(20, 20))
|
||||
|
||||
def get_statistics(self, all_sprites):
|
||||
###Garbage collector stats###
|
||||
gc_taken_space_plastic = 0
|
||||
gc_taken_space_metal = 0
|
||||
gc_taken_space_glass = 0
|
||||
gc_trash_space = 10
|
||||
|
||||
###Board stats###############
|
||||
plastic_left = 0
|
||||
metal_left = 0
|
||||
glass_left = 0
|
||||
total_gathered = 0
|
||||
|
||||
for item in all_sprites:
|
||||
if(type(item) == House):
|
||||
rubbish = item.get_rubbish_data()
|
||||
plastic_left += rubbish[0]
|
||||
glass_left += rubbish[1]
|
||||
metal_left += rubbish[2]
|
||||
if(type(item) == Garbage_collector):
|
||||
space_taken = item.get_space_data()
|
||||
gc_taken_space_plastic += space_taken.get("plastic")
|
||||
gc_taken_space_glass += space_taken.get("glass")
|
||||
gc_taken_space_metal += space_taken.get("metal")
|
||||
total_gathered += item.get_collect_data()
|
||||
|
||||
print("plastic left: "+str(plastic_left)+" | glass left: "+str(glass_left)+" | metal left: "+str(metal_left))
|
||||
print(" plastic: "+str(gc_taken_space_plastic)+"/"+str(gc_trash_space)+" | glass: "+str(gc_taken_space_glass)+"/"+str(gc_trash_space)+" | metal: "+str(gc_taken_space_metal)+"/"+str(gc_trash_space))
|
||||
print("### TOTAL COLLECTED: "+str(total_gathered)+" ###")
|
11
sprites/landfill.py
Normal file
@ -0,0 +1,11 @@
|
||||
import pygame
|
||||
import sys
|
||||
from sprites.cell import Cell
|
||||
|
||||
|
||||
class Landfill(Cell):
|
||||
def __init__(self, x, y, type):
|
||||
Cell.__init__(self, x, y)
|
||||
types = ["plastic", "glass", "metal"]
|
||||
self.type = types[type]
|
||||
self.image = pygame.image.load("images/landfill_%s.png" % (self.type))
|
6
to_do.txt
Normal file
@ -0,0 +1,6 @@
|
||||
+Przy losowaniu domku: Sprawdzić, czy pole które wylosowaliśmy jest typu Grass (bo nie można go postawić na wysypisku ani na innym domku)
|
||||
+Dodanie metody od zapełniania śmietników która updatuje się co klatkę (Jeżeli zapełnienie == 100 to zmienia się sprite i trzeba zabrać czy coś takiego)
|
||||
-Dodanie hudu
|
||||
+Wpisywanie na początku gry liczby domków
|
||||
+Umieszczenie na mapie wysypisk(I dodanie ich klasy)
|
||||
-W JAKI SPOSÓB MOŻNA PREZENTOWAĆ KILKA RÓŻNYCH ŚMIECI NA DOMKU?
|
53
utilities.py
@ -1,53 +0,0 @@
|
||||
from config import GRID_WIDTH, GRID_HEIGHT
|
||||
from DataModels.Road import Road
|
||||
import json, os, platform
|
||||
|
||||
def movement(environment, x ,y):
|
||||
movement = {
|
||||
"right": (x + 1, y) if x + 1 < GRID_WIDTH and type(environment[x + 1][y]) == Road else (x, y),
|
||||
"left": (x - 1, y) if x - 1 >= 0 and type(environment[x - 1][y]) == Road else (x, y),
|
||||
"down": (x, y + 1) if y + 1 < GRID_HEIGHT and type(environment[x][y + 1]) == Road else (x, y),
|
||||
"up": (x, y - 1) if y - 1 >= 0 and type(environment[x][y - 1]) == Road else (x, y)
|
||||
}
|
||||
|
||||
forbidden_movement = {
|
||||
"right": "left",
|
||||
"left": "right",
|
||||
"up": "down",
|
||||
"down": "up"
|
||||
}
|
||||
|
||||
return (movement, forbidden_movement)
|
||||
|
||||
def check_moves(environment, x,y,direction=None):
|
||||
if direction == None:
|
||||
return ([dir for dir in movement(environment, x, y)[0] if movement(environment, x,y)[0][dir] != (x,y)])
|
||||
return ([dir for dir in movement(environment, x, y)[0] if movement(environment, x,y)[0][dir] != (x,y) and dir != movement(environment,x,y)[1][direction]])
|
||||
|
||||
def save_moveset(moveset):
|
||||
if platform.system() == 'Windows':
|
||||
path = '\moveset_data.json'
|
||||
else:
|
||||
path = '/moveset_data.json'
|
||||
output_file = os.path.normpath(os.getcwd()) + path
|
||||
|
||||
results = {}
|
||||
try:
|
||||
f = open(output_file, 'r+')
|
||||
except:
|
||||
open(output_file, 'a').close()
|
||||
finally:
|
||||
f = open(output_file, 'r+')
|
||||
|
||||
try:
|
||||
results = json.load(f)
|
||||
except:
|
||||
pass
|
||||
finally:
|
||||
if "moveset" not in results:
|
||||
results = { "moveset": [] }
|
||||
|
||||
results["moveset"].append(moveset)
|
||||
f.seek(0)
|
||||
json.dump(results, f, indent=1)
|
||||
f.close()
|
71
utils.py
Normal file
@ -0,0 +1,71 @@
|
||||
import sys
|
||||
import getopt
|
||||
import random
|
||||
from config import PLAY_WIDTH, PLAY_HEIGHT, home_amount
|
||||
from sprites.cell import CELL_SIZE
|
||||
from sprites.grass import Grass
|
||||
from sprites.house import House
|
||||
from sprites.landfill import Landfill
|
||||
from sprites.garbage_collector import Garbage_collector
|
||||
|
||||
|
||||
def generate_rand_coordinates(max_x, max_y):
|
||||
return (random.randint(0, max_x), random.randint(0, (max_y)))
|
||||
|
||||
##GENERATE GRASS##################################################################
|
||||
def generate_grass(all_sprites):
|
||||
grass = []
|
||||
for k in range(0, (PLAY_WIDTH//CELL_SIZE)*(PLAY_HEIGHT//CELL_SIZE)):
|
||||
x, y = (int(k % (PLAY_WIDTH//CELL_SIZE)),
|
||||
int(k/(PLAY_WIDTH//CELL_SIZE)))
|
||||
grass.append(Grass(x, y))
|
||||
|
||||
for item in grass:
|
||||
all_sprites.add(item)
|
||||
##################################################################################
|
||||
|
||||
##GENERATE HOUSES#################################################################
|
||||
|
||||
|
||||
def generate_houses(all_sprites, obstacles_coords):
|
||||
houses = []
|
||||
home_counter = home_amount
|
||||
while(home_counter != 0):
|
||||
x, y = generate_rand_coordinates(
|
||||
(PLAY_WIDTH//CELL_SIZE)-1, (PLAY_HEIGHT//CELL_SIZE)-1)
|
||||
if(((x, y)) not in obstacles_coords["homes"] and ((x, y)) not in obstacles_coords["landfills"] and ((x, y-1)) not in obstacles_coords["landfills"]):
|
||||
houses.append(House(x, y, 10, 10, 10))
|
||||
obstacles_coords["homes"].append((x, y))
|
||||
home_counter = home_counter - 1
|
||||
|
||||
for item in houses:
|
||||
all_sprites.add(item)
|
||||
##################################################################################
|
||||
|
||||
##GENERATE LANDFILLS##############################################################
|
||||
|
||||
|
||||
def generate_landfills(all_sprites, obstacles_coords):
|
||||
landfills = []
|
||||
landfill_counter = 3
|
||||
y=0
|
||||
for x in range(landfill_counter):
|
||||
landfills.append(Landfill(x,y,x))
|
||||
obstacles_coords["landfills"].append((x,y))
|
||||
for item in landfills:
|
||||
all_sprites.add(item)
|
||||
##################################################################################
|
||||
|
||||
##GENERATE GARBAGE COLLECTOR######################################################
|
||||
|
||||
|
||||
def generate_garbage_collector(all_sprites, obstacles_coords):
|
||||
while(True):
|
||||
x, y = generate_rand_coordinates(
|
||||
(PLAY_WIDTH//CELL_SIZE)-1, (PLAY_HEIGHT//CELL_SIZE)-1)
|
||||
if((x, y) not in obstacles_coords["landfills"] and (x, y) not in obstacles_coords["homes"]):
|
||||
gc = Garbage_collector(x, y)
|
||||
break
|
||||
all_sprites.add(gc)
|
||||
return gc
|
||||
##################################################################################
|